#include<iostream> using namespace std; class shape { public: virtual float GetAre() = 0; }; class circle :public shape { public: circle(); circle(float R) { r = R; }; float GetAre(); private: float r; }; class rectangle :public shape { public: rectangle(); rectangle(float L, float W) { l = L; w = W; }; float GetAre(); private: float l, w; }; class square :public rectangle { public: square(float A) { a = A; }; float GetAre(); private: float a; }; float circle::GetAre() { return 3.14 * r * r; } //calculate the area of circle float rectangle::GetAre() { return l * w; } //calculate the area of rectangle float square::GetAre() { return a * a; } //calculate the area of square int main() { shape* p; p = new circle(2); cout << "The area of the circle(2) is" << p->GetAre() << endl; p = new rectangle(2, 3); cout << "The area of the rectangle(2,3) is" << p->GetAre() << endl; p = new square(2); cout << "The area of the square(2) is" << p->GetAre() << endl; return 0; }这个程序显示有一个无法解析的外部指令,这该如何修改
时间: 2024-03-10 08:45:38 浏览: 15
这个错误可能是由于缺少头文件或编译器无法链接到所需的库文件引起的。请尝试检查程序中是否缺少头文件或库文件,并确保编译器能够正确地链接到所需的库文件。
同时,建议将程序中的构造函数实现添加上去,可以避免一些潜在的问题。例如:
```c++
class circle : public shape {
public:
circle() { r = 0; }
circle(float R) { r = R; }
float GetAre();
private:
float r;
};
class rectangle : public shape {
public:
rectangle() { l = w = 0; }
rectangle(float L, float W) { l = L; w = W; }
float GetAre();
private:
float l, w;
};
class square : public rectangle {
public:
square() { a = 0; }
square(float A) { a = A; l = w = a; }
float GetAre();
private:
float a;
};
```
另外,建议在使用完指针后,释放动态分配的内存,避免内存泄漏。可以在main函数的最后添加以下代码:
```c++
delete p;
```
这样就可以释放动态分配的内存。完整的程序代码如下:
```c++
#include<iostream>
using namespace std;
class shape {
public:
virtual float GetAre() = 0;
};
class circle : public shape {
public:
circle() { r = 0; }
circle(float R) { r = R; }
float GetAre();
private:
float r;
};
class rectangle : public shape {
public:
rectangle() { l = w = 0; }
rectangle(float L, float W) { l = L; w = W; }
float GetAre();
private:
float l, w;
};
class square : public rectangle {
public:
square() { a = 0; }
square(float A) { a = A; l = w = a; }
float GetAre();
private:
float a;
};
float circle::GetAre() {
return 3.14 * r * r;
}
float rectangle::GetAre() {
return l * w;
}
float square::GetAre() {
return a * a;
}
int main() {
shape* p;
p = new circle(2);
cout << "The area of the circle(2) is" << p->GetAre() << endl;
delete p;
p = new rectangle(2, 3);
cout << "The area of the rectangle(2,3) is" << p->GetAre() << endl;
delete p;
p = new square(2);
cout << "The area of the square(2) is" << p->GetAre() << endl;
delete p;
return 0;
}
```
相关推荐
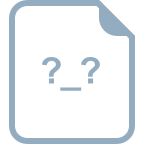














