用Dijkstra算法表示第一行包含两个正整数 N,MN,M (1\le N\le 150,\ 1\le M\le 1000)(1≤N≤150, 1≤M≤1000),分别代表现有城镇的数目和已修建的道路的数目。城镇分别以 0\sim N-10∼N−1 编号。 接下来是 MM 行道路信息。每一行有三个整数 A,B,XA,B,X (0\le A,B\lt N,\ A\ne B,\ 0\lt X\lt 10000)(0≤A,B<N, A =B, 0<X<10000),表示城镇 AA 和城镇 BB 之间有一条长度为 XX 的双向道路。 最后一行有两个整数 S,TS,T (0\le S,T\lt N)(0≤S,T<N),分别代表起点和终点。
时间: 2023-11-27 21:05:26 浏览: 104
下面是使用Dijkstra算法求解最短路径的示例代码,假设数据已经读入到邻接表adj和边权数组cost中。
```
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
const int INF = 0x3f3f3f3f;
vector<int> adj[150];
int cost[150][150];
int dist[150];
bool visited[150];
void dijkstra(int start, int end, int n) {
for (int i = 0; i < n; i++) {
dist[i] = INF;
visited[i] = false;
}
dist[start] = 0;
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
pq.push({0, start});
while (!pq.empty()) {
int u = pq.top().second;
pq.pop();
if (visited[u])
continue;
visited[u] = true;
for (int v : adj[u]) {
if (dist[v] > dist[u] + cost[u][v]) {
dist[v] = dist[u] + cost[u][v];
pq.push({dist[v], v});
}
}
}
cout << dist[end] << endl;
}
int main() {
int n, m;
cin >> n >> m;
for (int i = 0; i < m; i++) {
int a, b, x;
cin >> a >> b >> x;
adj[a].push_back(b);
adj[b].push_back(a);
cost[a][b] = cost[b][a] = x;
}
int start, end;
cin >> start >> end;
dijkstra(start, end, n);
return 0;
}
```
在上面的代码中,我们使用了一个优先队列来维护当前未访问的节点集合,每次从中选取距离起点最近的节点,并更新其邻居节点的距离。visited数组用于标记每个节点是否已经被访问,dist数组用于存储起点到每个节点的最短距离。最后,输出dist[end]作为结果,即起点到终点的最短距离。
阅读全文
相关推荐
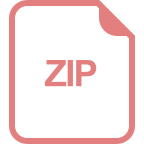
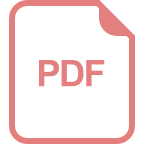
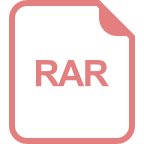















