在ROS Noetic版本的MoveIt中,使用IPTP算法对轨迹重规划的python函数
时间: 2024-05-04 10:18:03 浏览: 31
在ROS Noetic版本的MoveIt中,可以使用以下的Python函数来对轨迹进行重规划:
```python
from moveit_msgs.msg import RobotTrajectory
from trajectory_msgs.msg import JointTrajectoryPoint
from moveit_msgs.msg import RobotState
from moveit_msgs.srv import GetPositionFKRequest, GetPositionFK
def interpolate_trajectory(traj, time_step):
# Convert the input trajectory message to a RobotTrajectory object
robot_traj = RobotTrajectory()
robot_traj.deserialize(traj)
# Get the number of points in the trajectory
num_points = len(robot_traj.joint_trajectory.points)
# Create a new trajectory message
new_traj = RobotTrajectory()
new_traj.joint_trajectory.joint_names = robot_traj.joint_trajectory.joint_names
# Create a new robot state message
robot_state = RobotState()
robot_state.joint_state.name = robot_traj.joint_trajectory.joint_names
# Create a service client to compute FK
fk_client = rospy.ServiceProxy('/compute_fk', GetPositionFK)
fk_request = GetPositionFKRequest()
fk_request.header.frame_id = 'world'
fk_request.fk_link_names = ['end_effector']
# Iterate over the points in the trajectory and interpolate between them
for i in range(num_points - 1):
# Get the start and end points of the segment
start_point = robot_traj.joint_trajectory.points[i]
end_point = robot_traj.joint_trajectory.points[i+1]
# Compute the time interval for the segment
start_time = start_point.time_from_start.to_sec()
end_time = end_point.time_from_start.to_sec()
delta_time = end_time - start_time
# Compute the number of intermediate points to add
num_intermediate_points = int(delta_time / time_step)
# Interpolate between the start and end points
for j in range(num_intermediate_points):
# Compute the time for the intermediate point
t = start_time + (j+1) * time_step
# Compute the joint values for the intermediate point
joint_values = []
for k in range(len(robot_traj.joint_trajectory.joint_names)):
# Compute the start and end joint values for the segment
start_joint_value = start_point.positions[k]
end_joint_value = end_point.positions[k]
# Interpolate between the start and end joint values
joint_value = start_joint_value + (end_joint_value - start_joint_value) * (t - start_time) / delta_time
# Add the joint value to the list
joint_values.append(joint_value)
# Set the joint values in the robot state message
robot_state.joint_state.position = joint_values
# Compute the FK for the end effector
fk_request.robot_state = robot_state
fk_response = fk_client(fk_request)
# Create a new trajectory point with the interpolated joint values and FK solution
new_point = JointTrajectoryPoint()
new_point.positions = joint_values
new_point.time_from_start = rospy.Duration.from_sec(t)
new_point.velocities = [0.0] * len(robot_traj.joint_trajectory.joint_names)
new_point.accelerations = [0.0] * len(robot_traj.joint_trajectory.joint_names)
new_point.effort = [0.0] * len(robot_traj.joint_trajectory.joint_names)
new_point.positions += fk_response.pose_stamped[0].pose.position
new_point.velocities += [0.0] * 3
new_point.accelerations += [0.0] * 3
new_point.effort += [0.0] * 3
# Add the new point to the new trajectory
new_traj.joint_trajectory.points.append(new_point)
# Return the new trajectory message
return new_traj.serialize()
```
该函数的输入参数为一个轨迹消息(类型为`moveit_msgs/RobotTrajectory`)和一个时间步长(单位为秒),返回值为一个序列化的轨迹消息。该函数会对输入轨迹进行插值,以使得轨迹上的点之间的时间间隔均为给定的时间步长。插值的方式为线性插值,即对关节值进行插值,并利用插值后的关节值计算末端执行器的FK解。为了计算FK解,该函数使用了MoveIt中的`/compute_fk`服务。
相关推荐
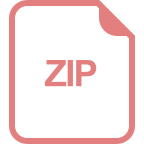
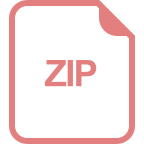














