Training Tips for YOLOv10: Secrets to Enhancing Model Performance and Facilitating Efficient Model Training
发布时间: 2024-09-13 20:39:40 阅读量: 31 订阅数: 23 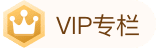
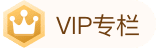
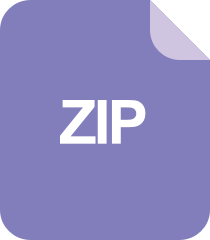
kubernetes-training:Kubernetes概念培训
# 1. Overview of YOLOv10 Training
As the latest breakthrough in the field of object detection, YOLOv10 is renowned for its outstanding accuracy and speed. Its training process involves several critical steps, including data preparation, model training, and evaluation. This chapter will outline the YOLOv10 training流程 to lay the foundation for in-depth exploration of specific techniques in subsequent chapters.
The first step in YOLOv10 training is to prepare the training data. This involves selecting an appropriate training dataset and preprocessing it, such as adjusting images and data augmentation. Data augmentation techniques, such as image flipping and cropping, help to increase the diversity of the training data and prevent model overfitting.
Next is the model training process. YOLOv10 utilizes advanced optimization algorithms, such as Adam, to minimize the loss function. Hyperparameters, such as learning rate and batch size, require careful adjustment to achieve optimal training results. Regularization techniques, such as Dropout and L2 regularization, help to prevent model overfitting and improve generalization capabilities.
# 2. YOLOv10 Training Tips
### 2.1 Data Augmentation Techniques
Data augmentation is a key technique to enhance the generalization and robustness of YOLOv10 models. By applying a series of transformations to the original images, new training samples are generated, thereby increasing the diversity of the model's training data.
#### 2.1.1 Image Flipping and Rotation
Image flipping and rotation are common data augmentation techniques. They generate images with different directions and perspectives, helping the model learn various object poses.
**Code Block:**
```python
import cv2
def flip_image(image, direction):
if direction == 'horizontal':
return cv2.flip(image, 1)
elif direction == 'vertical':
return cv2.flip(image, 0)
def rotate_image(image, angle):
return cv2.rotate(image, cv2.ROTATE_90_CLOCKWISE)
```
**Logical Analysis:**
* The `flip_image()` function flips the image horizontally or vertically based on the specified direction.
* The `rotate_image()` function rotates the image 90 degrees counterclockwise.
**Parameter Explanation:**
* `image`: Input image
* `direction`: Flipping direction ('horizontal' or 'vertical')
* `angle`: Rotation angle (in degrees)
#### 2.1.2 Image Cropping and Scaling
Image cropping and scaling can change the size and area of the image, helping the model to learn local features and different scales of objects.
**Code Block:**
```python
import cv2
def crop_image(image, x, y, w, h):
return image[y:y+h, x:x+w]
def resize_image(image, new_size):
return cv2.resize(image, new_size)
```
**Logical Analysis:**
* The `crop_image()` function crops a specified region from the image.
* The `resize_image()` function resizes the image to a specified new size.
**Parameter Explanation:**
* `image`: Input image
* `x`: Top-left x coordinate of the cropping region
* `y`: Top-left y coordinate of the cropping region
* `w`: Width of the cropping region
* `h`: Height of the cropping region
* `new_size`: New image size (tuple)
### 2.2 Hyperparameter Optimization
Hyperparameter optimization involves adjusting parameters during the model training process to achieve optimal performance. Key hyperparameters in YOLOv10 include learning rate, weight decay, batch size, and the number of training epochs.
#### 2.2.1 Learning Rate and Weight Decay
The learning rate controls the step size of the model's weight updates, while weight decay prevents overfitting of the model.
**Code Block:**
```python
import torch
optimizer = torch.optim.SGD(model.parameters(), lr=0.001, weight_decay=0.0005)
```
**Logical Analysis:**
* Uses stochastic gradient descent (SGD) optimizer.
* Sets the learning rate to 0.001.
* Sets the weight decay to 0.0005.
**Parameter Explanation:**
* `model.parameters()`: Model parameters
* `lr`: Learning rate
* `weight_decay`: Weight decay
#### 2.2.2 Batch Size and Number of Training Epochs
The batch size refers to the number of samples used in each training step, while the number of training epochs refers to the total number of iterations the model is trained for.
**Code Block:**
```python
batch_size = 32
num_epochs = 100
```
**Logical Analysis:**
* Sets the batch size to 32.
* Sets the number of training epochs to 100.
**Parameter Explanation:**
* `batch_size`: Batch size
* `num_epochs`: Number of training epochs
### 2.3 Model R***
***mon regularization techniques in YOLOv10 include Dropout and L2 regularization.
#### 2.3.1 Dropout and L2 Regularization
Dropout randomly drops neurons in the network, while L2 regularization adds a penalty term based on the size of the weights to the loss function.
**Code Block:**
```python
import torch.nn as nn
class DropoutLayer(nn.Module):
def __init__(self, p=0.5):
super(DropoutLayer, self).__init__()
self.p = p
def forward(self, x):
return nn.functional.dropout(x, self.p, training=self.training)
class L2Regularization(nn.Module):
def __init__(self, weight_decay):
super(L2Regularization, self).__init__()
self.weight_decay = weight_decay
def forward(self, model):
loss = 0
for param in model.parameters():
loss += self.weight_decay * torch.norm(param)
return loss
```
**Logical Analysi
0
0
相关推荐







