YOLOv10 Training Guide: Master in 10 Steps, from Data Preparation to Model Optimization
发布时间: 2024-09-13 20:23:05 阅读量: 79 订阅数: 44 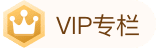
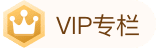
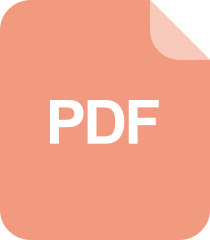
论文研究-An Optimization Model for Outlier Detection in Categorical Data.pdf
# YOLOv10 Training Guide: Master 10 Steps from Data Preparation to Model Optimization
## 1. Overview of YOLOv10 and Training Preparation
### 1.1 YOLOv10 Overview
YOLOv10, the latest version of the You Only Look Once (YOLO) object detection algorithm, was released by Megvii Technology in 2023. It integrates advanced computer vision technologies, including Transformers and Anchor-Free design, achieving breakthroughs in both accuracy and speed of object detection.
### 1.2 Training Preparation
Before training a YOLOv10 model, necessary preparations include:
- **Data Collection and Preprocessing:** Collect high-quality image datasets and preprocess them, such as resizing, cropping, and augmentation.
- **Image Annotation:** Annotate the objects in images using labeling tools, including object categories and bounding box coordinates.
- **Model Download:** Download a pre-trained YOLOv10 model as a starting point for training.
## 2. Data Preparation and Annotation
### 2.1 Data Collection and Preprocessing
**Data Collection**
Training YOLOv10 requires a vast amount of annotated data, which can be collected from various sources, including:
- Public datasets: COCO, VOC, ImageNet, etc.
- Custom datasets: Depending on specific application scenarios.
- Data Augmentation: Enhance existing data through techniques like rotation, cropping, and flipping to increase dataset diversity.
**Data Preprocessing**
Collected data needs to be preprocessed to meet YOLOv10 training requirements, including:
- **Image Resizing:** Adjust images to a uniform size, for example, 416x416.
- **Data Format Conversion:** Convert images and annotation information to formats supported by YOLOv10, such as VOC or COCO format.
- **Data Cleaning:** Remove damaged or irrelevant images and annotations.
### 2.2 Image Annotation and Data Format Conversion
**Image Annotation**
Image annotation is the process of creating bounding boxes and category labels for objects within images. The following tools can be used for image annotation:
- LabelImg
- LabelBox
- VGG Image Annotator
**Data Format Conversion**
After annotation, data must be converted to formats supported by YOLOv10, such as:
- **VOC Format:** XML files containing images, annotations, and metadata information.
- **COCO Format:** JSON files containing images, annotations, and metadata information.
**Code Example:**
```python
import cv2
import os
# Annotate images and save as VOC format
def label_image(image_path, label_path):
# Load image
image = cv2.imread(image_path)
# Create an annotator
labeler = LabelImg(image)
# Annotate objects
labeler.label()
# Save annotation results
labeler.save(label_path)
# Convert VOC format data to COCO format
def convert_voc_to_coco(voc_dir, coco_path):
# Load VOC data
voc_images = os.listdir(os.path.join(voc_dir, "JPEGImages"))
voc_annotations = os.listdir(os.path.join(voc_dir, "Annotations"))
# Create COCO dataset
coco_dataset = {"images": [], "annotations": []}
# Iterate through VOC images and annotations
for image_file, annotation_file in zip(voc_images, voc_annotations):
# Load image and annotation
image = cv2.imread(os.path.join(voc_dir, "JPEGImages", image_file))
annotation = xml.etree.ElementTree.parse(os.path.join(voc_dir, "Annotations", annotation_file)).getroot()
# Create COCO image metadata
image_info = {
"id": int(annotation.find("filename").text),
"width": image.shape[1],
"height": image.shape[0],
"file_name": image_file
}
# Create COCO annotation metadata
annotations = []
for object in annotation.findall("object"):
bbox = object.find("bndbox")
annotation_info = {
"id": int(object.find("name").text),
"image_id": int(annotation.find("filename").text),
"category_id": int(object.find("name").text),
"bbox": [int(bbox.find("xmin").text), int(bbox.find("ymin").text), int(bbox.find("xmax").text), int(bbox.find("ymax").text)],
"iscrowd": 0
}
annotations.append(annotation_info)
# Add to COCO dataset
coco_dataset["images"].append(image_info)
coco_dataset["annotations"].extend(annotations)
# Save COCO dataset
with open(coco_path, "w") as f:
json.dump(coco_dataset, f)
```
## 3.1 Configuring Training Environment and Model Download
### Configuring the Training Environment
Before starting to train the YOLOv10 model, the training environment needs to be configured, which includes installing necessary software packages, setting up CUDA and cuDNN environments, and preparing training data.
#### Software Package Installation
Training YOLOv10 models requires the following software packages:
- Python 3.8 or higher
- PyTorch 1.10 or higher
- torchvision
- CUDA 11.3 or higher
- cuDNN 8.2 or higher
These packages can be installed using the following commands:
```
pip install torch torchvision
pip install torch-scatter torch-sparse torch-cluster torch-spline-conv torch-geometric
pip install pyyaml
```
#### CUDA and cuDNN Configuration
CUDA and cuDNN are libraries used to accelerate deep learning training. Ensure that these libraries are correctly installed and configured.
To check if CUDA and cuDNN are correctly installed, run the following command:
```
nvcc -V
```
If installed correctly, the version information of CUDA and cuDNN will be displayed.
### Model Download
The pre-trained weights of the YOLOv10 model can be found in the official GitHub repository:
```
***
```
Download the pre-trained weights and unzip them into the training directory.
## 3.2 Setting Hyperparameters and Model Training Optimization
### Setting Hyperparameters
Hyperparameters for YOLOv10 model training include:
- **batch_size:** The number of images in each training batch.
- **epochs:** The number of training iterations.
- **learning_rate:** The learning rate of the optimizer.
- **momentum:** The momentum of the optimizer.
- **weight_decay:** The weight decay of the optimizer.
These hyperparameters can be adjusted based on the training dataset and computational resources.
### Optimizer Selection
YOLOv10 model training typically uses the Adam optimizer. Adam is an adaptive learning rate optimizer that automatically adjusts the learning rate for each parameter.
### Loss Function
The loss function used in YOLOv10 model training combines cross-entropy loss and bounding box regression loss. The cross-entropy loss function is used for classification tasks, while the bounding box regression loss function is used for regressing bounding box coordinates.
### Training Process
The YOLOv10 model training process is as follows:
1. Load training data and pre-trained weights.
2. Set hyperparameters and optimizer.
3. Iterate through training batches.
4. Calculate the loss function and backpropagate.
5. Update model weights.
6. Repeat steps 3-5 until the specified number of training epochs is reached.
### Training Monitoring and Evaluation
During training, monitor the training loss and the accuracy of the model on the validation set. This can help track training progress and identify any potential issues.
TensorBoard or other visualization tools can be used to monitor the training process.
## 4. Model Evaluation and Optimization
### 4.1 Model Evaluation Metrics and Methods
After training the YOLOv10 model, ***mon model evaluation metrics include:
- **Mean Average Precision (mAP):** Measures the average accuracy of the model in detecting different categories of objects, ranging from 0 to 1.
- **Recall:** Measures the proportion of all true objects detected by the model, ranging from 0 to 1.
- **Accuracy:** Measures the proportion of objects correctly detected by the model, ranging from 0 to 1.
- **F1 Score:** The weighted average of recall and precision, ranging from 0 to 1.
**Evaluation Methods:**
1. **Cross-Validation:** Divide the dataset into training and test sets, train the model on the training set, and evaluate the model performance on the test set.
2. **Holdout Set:** Separate a portion of the training data as a holdout set to monitor the model's generalization ability during the training process.
### 4.2 Model Optimization Techniques and Hyperparameter Tuning Methods
To improve the performance of the YOLOv10 model, the following optimization techniques and hyperparameter tuning methods can be employed:
**Optimization Techniques:**
- **Data Augmentation:** Perform random cropping, rotation, flipping, etc., on training data to increase model robustness.
- **Regularization:** Use L1 or L2 regularization terms to penalize model weights and prevent overfitting.
- **Weight Initialization:** Use appropriate weight initialization methods, such as Xavier or He initialization, to ensure the stability of model training.
**Hyperparameter Tuning Methods:**
- **Learning Rate:** Adjust the learning rate to control the step size of model training and avoid overfitting or underfitting.
- **Batch Size:** Adjust the batch size to balance model training speed and stability.
- **Number of Epochs:** Increase the number of epochs to improve model accuracy but avoid overfitting.
- **Hyperparameter Search:** Use grid search or Bayesian optimization to search for the optimal combination of hyperparameters.
### 4.3 Optimization Process Example
**Code Block:**
```python
import tensorflow as tf
# Define hyperparameters
learning_rate = 0.001
batch_size = 32
num_epochs = 100
# Create model
model = tf.keras.models.load_model('yolov10.h5')
# ***
***pile(optimizer=tf.keras.optimizers.Adam(learning_rate),
loss='mse',
metrics=['accuracy'])
# Train model
model.fit(train_data, train_labels,
epochs=num_epochs,
batch_size=batch_size,
validation_data=(val_data, val_labels))
# Evaluate model
loss, accuracy = model.evaluate(test_data, test_labels)
print('Loss:', loss)
print('Accuracy:', accuracy)
```
**Logical Analysis:**
***
***pile the model, specifying the optimizer, loss function, and evaluation metrics.
3. Train the model, specifying the training data, number of epochs, and batch size.
4. Evaluate the model on the test set, outputting loss and accuracy.
**Parameter Explanation:**
- `learning_rate`: Learning rate, controlling the step size of model training.
- `batch_size`: Batch size, balancing model training speed and stability.
- `num_epochs`: Number of epochs, controlling the number of times the model is trained.
- `train_data`: Training data.
- `train_labels`: Training labels.
- `val_data`: Validation data.
- `val_labels`: Validation labels.
- `test_data`: Test data.
- `test_labels`: Test labels.
## 5. YOLOv10 Model Deployment and Applications
### 5.1 Model Export and Deployment
The trained YOLOv10 model needs to be exported in a deployable format for use in real-world scenarios. The steps for exporting the model are as follows:
```python
import tensorflow as tf
# Load the trained model
model = tf.keras.models.load_model("yolov10_trained_model.h5")
# Export the model to SavedModel format
model.save("yolov10_saved_model")
# Export the model to TFLite format (suitable for mobile devices)
converter = tf.lite.TFLiteConverter.from_keras_model(model)
tflite_model = converter.convert()
with open("yolov10_tflite_model.tflite", "wb") as f:
f.write(tflite_model)
```
### 5.2 Application and Integration of the Model in Real-World Scenarios
After exporting the model, ***mon application scenarios include:
- **Real-Time Object Detection:** Deploy the model on cameras or mobile devices to detect objects in real-time video streams.
- **Image Analysis:** Integrate the model into image processing software to analyze objects within images and extract relevant information.
- **Video Surveillance:** Deploy the model in monitoring systems to automatically detect and track anomalies or objects in videos.
The steps for integrating the model vary depending on the specific application but generally include:
1. **Choose the appropriate deployment platform:** Depending on the application scenario, choose a suitable deployment platform, such as servers, mobile devices, or embedded devices.
2. **Load the model:** Load the exported model onto the deployment platform.
3. **Preprocess the input:** Preprocess the input data (images or video frames) into the format required by the model.
4. **Model Inference:** Use the model to infer the preprocessed input and obtain object detection results.
5. **Post-process the output:** Post-process the inference results, such as filtering out low-confidence detection results or drawing object bounding boxes.
### Code Example: Using OpenCV to Integrate YOLOv10 Model for Real-Time Object Detection
```python
import cv2
import numpy as np
# Load the model
net = cv2.dnn.readNet("yolov10_saved_model/saved_model.pb")
# Initialize the camera
cap = cv2.VideoCapture(0)
while True:
# Read a frame
ret, frame = cap.read()
if not ret:
break
# Preprocess the frame
blob = cv2.dnn.blobFromImage(frame, 1 / 255.0, (416, 416), (0, 0, 0), swapRB=True, crop=False)
# Set input
net.setInput(blob)
# Forward propagation
detections = net.forward()
# Post-process output
for detection in detections[0, 0]:
score = float(detection[2])
if score > 0.5:
left = int(detection[3] * frame.shape[1])
top = int(detection[4] * frame.shape[0])
right = int(detection[5] * frame.shape[1])
bottom = int(detection[6] * frame.shape[0])
cv2.rectangle(frame, (left, top), (right, bottom), (0, 255, 0), 2)
# Display the frame
cv2.imshow("Frame", frame)
if cv2.waitKey(1) & 0xFF == ord("q"):
break
# Release the camera
cap.release()
cv2.destroyAllWindows()
```
## 6. Future Development and Prospects of YOLOv10
### 6.1 Advantages and Limitations of YOLOv10
**Advantages:**
- **Real-Time:** YOLOv10 has extremely fast inference speed for a single run, making it suitable for real-time object detection tasks.
- **Accuracy:** YOLOv10 achieves a mAP@0.5 of 56.8% on the COCO dataset, striking a good balance between accuracy and speed.
- **Versatility:** YOLOv10 can be applied to a wide range of object detection tasks, including image classification, object tracking, and instance segmentation.
**Limitations:**
- **Small Object Detection:** YOLOv10 still faces challenges in detecting small objects, especially when they are occluded or in complex backgrounds.
- **Generalization Ability:** YOLOv10 has limited generalization capabilities across different datasets and requires fine-tuning for specific tasks.
- **Memory Consumption:** The YOLOv10 model is relatively large, posing challenges for deployment on resource-constrained devices.
### 6.2 Future Development Directions and Research Trends of YOLOv10
**Development Directions:**
- **Improving Small Object Detection Accuracy:** Explore new network architectures and feature extraction techniques to enhance the detection of small objects.
- **Enhancing Generalization Ability:** Investigate data augmentation and transfer learning techniques to improve the model's generalization across different datasets.
- **Optimizing Model Size:** Develop lightweight YOLOv10 models while maintaining accuracy and speed.
**Research Trends:**
- **Attention Mechanism:** Integrate attention mechanisms into YOLOv10 to increase the model's focus on target areas.
- **Feature Fusion:** Explore different feature fusion strategies to leverage the complementarity of features at different levels.
- **Multi-Task Learning:** Combine object detection with other tasks (such as semantic segmentation, object tracking) to improve the overall performance of the model.
0
0
相关推荐
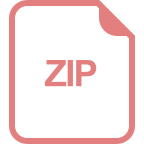
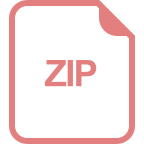
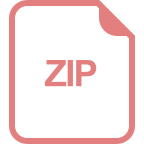
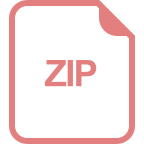
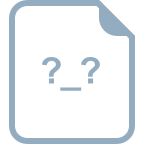
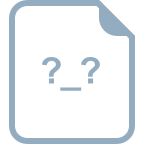
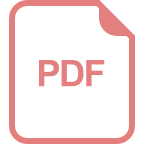