使用c++代码使mavros以0.3m/s的速度起飞后悬停
时间: 2023-06-17 08:03:38 浏览: 69
要使用C++代码使mavros起飞并悬停,你需要使用mavros库和ROS(Robot Operating System)。
以下是一个简单的代码示例,可以让mavros起飞并悬停:
```cpp
#include <ros/ros.h>
#include <mavros_msgs/CommandBool.h>
#include <mavros_msgs/CommandTOL.h>
#include <mavros_msgs/SetMode.h>
#include <geometry_msgs/TwistStamped.h>
int main(int argc, char **argv)
{
ros::init(argc, argv, "mavros_takeoff_and_hover");
ros::NodeHandle nh;
// 发布器和服务客户端
ros::Publisher velocity_pub = nh.advertise<geometry_msgs::TwistStamped>("/mavros/setpoint_velocity/cmd_vel", 10);
ros::ServiceClient arming_client = nh.serviceClient<mavros_msgs::CommandBool>("/mavros/cmd/arming");
ros::ServiceClient takeoff_client = nh.serviceClient<mavros_msgs::CommandTOL>("/mavros/cmd/takeoff");
ros::ServiceClient set_mode_client = nh.serviceClient<mavros_msgs::SetMode>("/mavros/set_mode");
// 等待连接到mavros
ros::Rate rate(20.0); // 设置循环频率为20hz
while (ros::ok() && !ros::service::waitForService("/mavros/set_mode", 1000)) {
ROS_INFO("等待连接到mavros...");
}
// 设置起飞高度
double takeoff_height = 3.0;
// 设置飞行模式
mavros_msgs::SetMode offb_set_mode;
offb_set_mode.request.custom_mode = "OFFBOARD";
// 设置起飞命令
mavros_msgs::CommandTOL takeoff_cmd;
takeoff_cmd.request.altitude = takeoff_height;
// 设置武器状态
mavros_msgs::CommandBool arm_cmd;
arm_cmd.request.value = true;
// 等待mavros就绪
while (ros::ok() && !arming_client.call(arm_cmd) && arm_cmd.response.success) {
ROS_WARN("武器状态设置失败,重试中...");
ros::Duration(1.0).sleep();
}
// 等待mavros就绪
while (ros::ok() && !takeoff_client.call(takeoff_cmd) && takeoff_cmd.response.success) {
ROS_WARN("起飞命令发送失败,重试中...");
ros::Duration(1.0).sleep();
}
// 等待mavros就绪
while (ros::ok() && !set_mode_client.call(offb_set_mode) && offb_set_mode.response.mode_sent) {
ROS_WARN("模式设置失败,重试中...");
ros::Duration(1.0).sleep();
}
// 悬停
geometry_msgs::TwistStamped velocity_cmd;
velocity_cmd.twist.linear.x = 0.0;
velocity_cmd.twist.linear.y = 0.0;
velocity_cmd.twist.linear.z = 0.0;
velocity_cmd.twist.angular.x = 0.0;
velocity_cmd.twist.angular.y = 0.0;
velocity_cmd.twist.angular.z = 0.0;
while (ros::ok()) {
velocity_pub.publish(velocity_cmd);
ros::spinOnce();
rate.sleep();
}
return 0;
}
```
这个代码使用了mavros库,通过发布速度话题来控制飞行器的运动。在起飞后,它发送了一个速度命令,使飞行器悬停在空中。
相关推荐
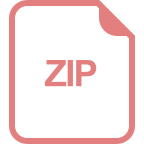














