osg使用NodeVisitor对遍历到的子节点进行旋转、平移的代码
时间: 2024-04-18 12:31:30 浏览: 34
在 OpenSceneGraph (OSG) 中,您可以使用 `NodeVisitor` 类来遍历场景图的节点,并对每个子节点进行旋转和平移操作。下面是一个示例代码片段,展示了如何使用 `NodeVisitor` 来对子节点进行旋转和平移:
```cpp
#include <osg/NodeVisitor>
#include <osg/MatrixTransform>
#include <osg/Geode>
#include <osgDB/ReadFile>
// 自定义的 NodeVisitor 子类
class MyVisitor : public osg::NodeVisitor
{
public:
MyVisitor() : osg::NodeVisitor(osg::NodeVisitor::TRAVERSE_ALL_CHILDREN) {}
// 重写 apply 方法,在遍历每个节点时执行操作
virtual void apply(osg::Node& node)
{
// 在这里添加旋转和平移的代码
osg::MatrixTransform* transform = dynamic_cast<osg::MatrixTransform*>(&node);
if (transform)
{
// 旋转节点
osg::Matrix rotationMatrix;
rotationMatrix.makeRotate(osg::Quat(osg::PI / 4, osg::Vec3(0, 0, 1))); // 绕 Z 轴旋转 45 度
transform->setMatrix(transform->getMatrix() * rotationMatrix);
// 平移节点
osg::Matrix translationMatrix;
translationMatrix.makeTranslate(osg::Vec3(1, 0, 0)); // 沿 X 轴平移 1 单位
transform->setMatrix(transform->getMatrix() * translationMatrix);
}
// 继续遍历子节点
traverse(node);
}
};
int main()
{
// 加载场景文件
osg::ref_ptr<osg::Node> scene = osgDB::readNodeFile("path/to/your/scene.osg");
// 创建 MyVisitor 对象并应用于场景节点
MyVisitor visitor;
scene->accept(visitor);
// 运行场景图的渲染循环...
return 0;
}
```
在上面的示例中,我们首先定义了一个名为 `MyVisitor` 的自定义 `NodeVisitor` 子类。在 `apply` 方法中,我们使用 `dynamic_cast` 操作将节点转换为 `osg::MatrixTransform` 类型,然后对其进行旋转和平移操作。然后,我们调用 `traverse` 方法继续遍历子节点。
在 `main` 函数中,我们加载场景文件并创建了 `MyVisitor` 对象,并将其应用于场景节点。然后,您可以根据需要执行场景图的渲染循环。
请注意,上述示例中的旋转和平移操作仅作为演示。您可以根据实际需求修改旋转和平移的参数。同时,您可能需要添加其他条件来筛选出特定的节点进行操作。
希望对您有所帮助!如果您有任何进一步的问题,请随时提问。
相关推荐
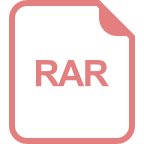
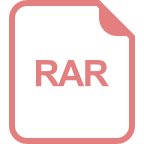
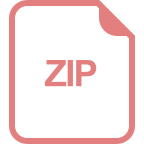














