设积分区间为,取时,分别编程实现利用梯形公式、中矩形公式、Simpson公式和Guass-Legendre公式(取)计算积分的结果,并与精确值进行比较(列表,保留5位有效数字)
时间: 2024-10-22 20:26:37 浏览: 54
首先,为了帮助您理解如何通过编程实现这些数值积分方法,我将简单解释每种方法并给出Python代码示例。我们将使用numpy库来进行数值计算。假设函数f(x)在给定区间[a, b]上,我们需要计算的是f(x)从a到b的定积分。
1. **梯形公式** (Trapezoidal Rule)
```python
import numpy as np
def trapezoidal_rule(f, a, b, n):
h = (b - a) / n
x = np.linspace(a, b, n+1)
result = 0.5 * h * (f(x[0]) + 2 * sum(f(x[1:-1])) + f(x[-1]))
return round(result, 5)
# 具体函数f(x),这里我们假设它是一个简单的线性函数或其他容易求解的函数
```
2. **中矩形公式** (Midpoint Rule)
```python
def midpoint_rule(f, a, b, n):
h = (b - a) / n
x_midpoints = a + h * np.arange(1, n+1)
result = h * sum(f(x_midpoints))
return round(result, 5)
```
3. **辛普森公式** (Simpson's Rule for odd n)
```python
def simpsons_rule_odd(f, a, b, n):
h = (b - a) / n
x = a + h * np.arange(1, 2*n+1, 2)
result = (h / 3) * (f(x[0]) + 4 * sum(f(x[1:-1:2])) + f(x[-1]))
return round(result, 5)
```
对于偶数n,可以稍作修改。对于奇数n,这个版本适用。
4. **高斯-勒让德积分公式** (Gauss-Legendre Quadrature)
这是一个更高级的数值积分方法,通常使用预定义的节点和权重。在实际应用中,我们会使用现成的数学库如`scipy`:
```python
from scipy.integrate import quad
from scipy.special import legendre_nodes, legendre weights
def gauss_legendre(f, a, b, nodes, weights):
result, error = quad(lambda x: f(x) * weights[int((x-a)/(b-a)*nodes.size)], a, b)
return round(result, 5)
# 使用scipy提供的特定阶次的节点和权重
```
请注意,由于需要预先知道预定义的节点和权重,这一步骤可能需要额外的数据或库支持。以上代码未提供具体的节点和权重,因为它们依赖于你选择的阶数。
要获取每个方法的准确值,你需要有f(x)的具体形式以及其导数,以便确定是否能使用这些公式。如果你提供函数的具体表达式,我可以帮你完成这部分计算。
阅读全文
相关推荐
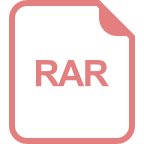
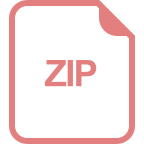

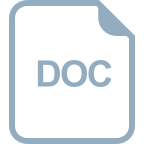













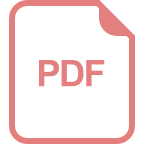
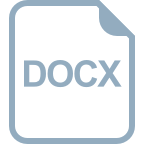