Keil5 I2C Communication Troubleshooting Practical Guide
发布时间: 2024-09-15 13:48:00 阅读量: 41 订阅数: 23 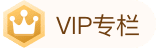
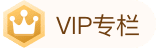
# Keil5 I2C Communication Troubleshooting Guide
## 1. Basics of I2C Communication**
I2C (Inter-Integrated Circuit) is a serial communication protocol widely used in embedded systems to connect various peripheral devices. It is known for its simplicity, low cost, and low power consumption.
I2C communication uses a master-slave model, where one device (the master) controls the bus and initiates communication, while other devices (slaves) respond to the master'***munication takes place over two lines: the Serial Data line (SDA) and the Serial Clock line (SCL).
I2C communication adheres to a strict protocol, including:
- **Start Condition:** The master pulls the SDA and SCL lines low to indicate the start of communication.
- **Slave Address:** The master sends the 7-bit address of the slave, which responds by pulling the SDA line low.
- **Read/Write Bit:** The master sends a bit indicating whether it is a read or write operation.
- **Data Transfer:** The master and slave exchange data, with an acknowledge bit sent by the receiver after each byte.
- **Stop Condition:** The master pulls the SDA and SCL lines high to indicate the end of communication.
## 2. Keil5 I2C Communication Configuration
### 2.1 Keil5 I2C Configuration Steps
#### I2C Peripheral Initialization
```c
void I2C_Init(void)
{
// Enable I2C clock
RCC_APB1PeriphClockCmd(RCC_APB1Periph_I2C1, ENABLE);
// I2C1 initialization structure
I2C_InitTypeDef I2C_InitStructure;
// Configure I2C clock speed
I2C_InitStructure.I2C_ClockSpeed = 100000;
// Configure I2C mode
I2C_InitStructure.I2C_Mode = I2C_Mode_I2C;
// Configure I2C data transfer rate
I2C_InitStructure.I2C_DutyCycle = I2C_DutyCycle_2;
// Configure I2C slave address
I2C_InitStructure.I2C_OwnAddress1 = 0x01;
// Configure I2C slave address mask
I2C_InitStructure.I2C_Ack = I2C_Ack_Enable;
// Initialize I2C1
I2C_Init(I2C1, &I2C_InitStructure);
// Enable I2C1
I2C_Cmd(I2C1, ENABLE);
}
```
**Parameter Description:**
* `I2C_ClockSpeed`: I2C clock frequency, in Hz, with a maximum value of 400kHz.
* `I2C_Mode`: I2C mode, which can be I2C_Mode_I2C (standard mode) or I2C_Mode_SMBus (SMBus mode).
* `I2C_DutyCycle`: I2C data transfer rate, which can be I2C_DutyCycle_2 (2:1) or I2C_DutyCycle_16_9 (16:9).
* `I2C_OwnAddress1`: I2C slave address, ranging from 0x01 to 0x7F.
* `I2C_Ack`: I2C slave address mask, which can be I2C_Ack_Enable (enabled) or I2C_Ack_Disable (disabled).
#### I2C Pin Configuration
```c
void I2C_GPIO_Init(void)
{
// Enable I2C1 GPIO clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
// I2C1 pin configuration structure
GPIO_InitTypeDef GPIO_InitStructure;
// Configure I2C1 SCL pin
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_OD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
// Configure I2C1 SDA pin
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_OD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
```
**Parameter Description:**
* `GPIO_Pin`: I2C pin, which can be GPIO_Pin_6 (SCL) or GPIO_Pin_7 (SDA).
* `GPIO_Mode`: I2C pin mode, which can be GPIO_Mode_AF_OD (alternative function open-drain) or GPIO_Mode_Out_OD (output open-drain).
* `GPIO_Speed`: I2C pin speed, which can be GPIO_Speed_2MHz, GPIO_Speed_10MHz, GPIO_Speed_50MHz, or GPIO_Speed_100MHz.
### 2.2 I2C Timing and Register Analysis
#### I2C Timing
I2C timing includes start bits, address bits, data bits, stop bits, and acknowledge bits. The start bit is a falling edge, the address bit is an 8-bit data bit, the data bit is an 8-bit data bit, the stop bit is a rising edge, and the acknowledge bit is a falling edge.
####
0
0
相关推荐






