Practical Guide to Writing and Optimizing Code with Keil5
发布时间: 2024-09-15 13:34:13 阅读量: 34 订阅数: 46 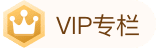
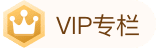
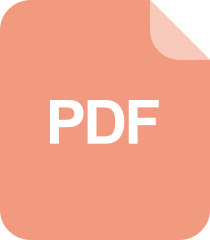
Red Hat Enterprise Linux 5 Tuning and Optimizing Red Hat Enterpr
# Practical Strategies for Optimizing Code with Keil 5
## 2.1 Principles and Methods of Code Optimization
### 2.1.1 Algorithm Opti***
***mon methods of algorithm optimization include:
- **Time Complexity Optimization:** Opting for algorithms with lower time complexity, such as using binary search over linear search.
- **Space Complexity Optimization:** Reducing the memory space required by algorithms, such as employing dynamic programming instead of recursion.
- **Parallelization:** Breaking down algorithms into multiple parallel tasks to increase efficiency.
### 2.1.2 Data Structure Optimiz***
***mon optimization methods include:
- **Choosing Suitable Data Structures:** Select the most fitting data structure based on the algorithm's needs, such as using a hash table over an array for quick lookups.
- **Optimizing Data Structures:** Enhance data structures by optimizing, such as using a self-balancing binary tree to improve query efficiency.
- **Reducing Data Redundancy:** Eliminate redundancy within data, such as using reference counting to avoid duplicating the same data storage.
# 2. Keil5 Code Optimization Techniques
### 2.1 Principles and Methods of Code Optimization
#### ***
***mon algorithm optimization methods include:
- **Time Complexity Optimization:** Lowering the time complexity of algorithms to reduce execution time.
- **Space Complexity Optimization:** Decreasing the space complexity to minimize memory usage.
- **Parallel Optimization:** Utilize multi-core processors or multi-threading technologies to divide tasks into subtasks that execute in parallel, enhancing code efficiency.
#### 2.1.2 Data Stru***
***mon methods include:
- **Choosing Suitable Data Structures:** Select the most appropriate data structure based on the characteristics of the data, such as arrays, linked lists, hash tables, etc.
- **Optimizing Storage Methods:** Adjust the storage method of data structures to reduce memory usage and enhance access efficiency.
- **Reducing Data Redundancy:** Avoid storing the same data repeatedly in code by using references or shared data structures to minimize redundancy.
### 2.2 Utilizing Keil5 Optimization Tools
#### 2.2.1 Keil5 Compiler Options
The Keil5 compiler offers various optimization options, such as:
- **Optimization Levels:** Specify the compiler's optimization level, ranging from no optimization to the highest level.
- **Code Generation Options:** Control options related to code generation, such as inlining functions and loop unrolling.
- **Floating-Point Handling Options:** Determine the method of handling floating-point operations, like precision and rounding modes.
#### 2.2.2 Keil5 Debugger Features
The Keil5 debugger provides multiple features to aid developers in analyzing code performance and identifying optimization points.
- **Performance Analysis:** Developers can understand code execution time and memory usage through performance analysis tools, thereby identifying performance bottlenecks.
- **Code Coverage Analysis:** With code coverage analysis tools, developers can see which parts of the code have been executed and which have not, discovering untested code paths.
- **Memory Analysis:** Through memory analysis tools, developers can assess memory allocation within the code, finding issues like memory leaks or fragmentation.
**Code Block:**
```c
// Before optimization
int sum(int n) {
int i, s = 0;
for (i = 1; i <= n; i++) {
s += i;
}
return s;
}
// After optimization
int sum(int n) {
return n * (n + 1) / 2;
}
```
**Logical Analysis:**
The optimized code employs the mathematical formula `n * (n + 1) / 2` to calculate the sum, avoiding the loop and greatly enhancing efficiency.
**Parameter Explanation:**
- `n`: The positive integer for which the sum is calculated.
# 3. Keil5 Code Optimization Practices
### 3.1 Common Optimization Issues in Embedded Systems
#### 3.1.1 Performance Bottleneck Analysis
Common performance bottlenecks in embedded systems include:
- **High CPU Utilization:** The CPU's execution time exceeds its available time, causing slow system responses.
- **Insufficient Memory:** Programs or data exceed available memory, r
0
0
相关推荐
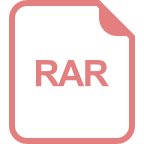
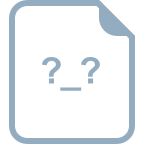
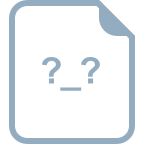
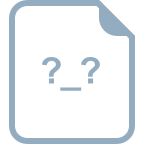
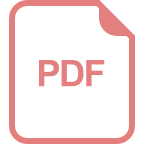
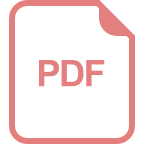
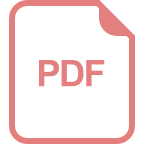
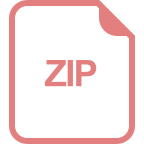