Keil5 Multitasking Scheduling: A Practical Guide to FreeRTOS
发布时间: 2024-09-15 13:40:11 阅读量: 38 订阅数: 50 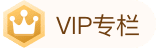
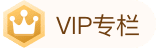
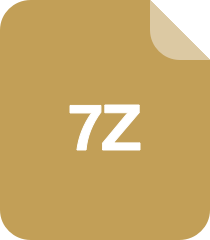
KEIL5编译器安装包:Compiler-506-Windows-x86-b960

# 1. Overview of Keil5 Multitasking Scheduling
Multitasking scheduling is an operating system technique that allows multiple tasks to run simultaneously within the same timeframe. In Keil5, FreeRTOS is a popular real-time operating system (RTOS) used for multitasking scheduling. FreeRTOS offers a rich set of APIs that enable developers to create and manage tasks, queues, semaphores, and timers. With FreeRTOS, developers can create complex multitasking applications that can efficiently utilize the resources of microcontrollers.
# 2. FreeRTOS Basic Concepts and Configuration
### 2.1 Introduction to FreeRTOS and Its Architecture
#### 2.1.1 Tasks, Queues, and Semaphores in FreeRTOS
FreeRTOS is a lightweight, preemptive, real-time operating system mainly used in embedded systems. It provides a series of core functionalities, including task management, synchronization and communication mechanisms, as well as timer management.
***Task:** A task is the smallest unit of execution in FreeRTOS, with each task having its own stack space and execution code. Tasks can run concurrently and can have different priorities.
***Queue:** A queue is a FIFO (first-in, first-out) data structure used for passing data between tasks. Tasks can put data into a queue or retrieve data from it.
***Semaphore:** A semaphore is a synchronization mechanism used to coordinate task access. Tasks can acquire a semaphore to indicate that a resource is available, or release a semaphore to allow other tasks to use the resource.
#### 2.1.2 FreeRTOS Priorities and Scheduling Algorithm
Tasks in FreeRTOS have priorities, with higher-priority tasks having more execution rights than lower-priority ones. FreeRTOS uses a preemptive scheduling algorithm, meaning higher-priority tasks can preempt lower-priority ones.
The FreeRTOS scheduling algorithm is based on the following principles:
***Ready Queue:** All ready tasks are stored in the ready queue, sorted by priority from high to low.
***Time Slice:** Each task has a time slice, and it will continue to execute until its time slice expires.
***Preemption:** If a higher-priority task becomes ready, it will preempt the currently executing task.
### 2.2 Configuring FreeRTOS in Keil5
#### 2.2.1 Creating a FreeRTOS Project
Creating a FreeRTOS project in Keil5 involves the following steps:
1. Open Keil5, select "File" -> "New" -> "uVision Project".
2. Enter the project name in "Project Name" and select the target device in "Target".
3. In the "Device" tab, select "CMSIS Device Database" and search for the target device.
4. In the "CMSIS Pack Manager," search for "FreeRTOS" and install the FreeRTOS library.
5. In the "Project" tab, check "Enable CMSIS-Pack" and select the FreeRTOS library.
6. Click "OK" to create the project.
#### 2.2.2 Configuring the FreeRTOS Kernel and Tasks
Configuring the FreeRTOS kernel and tasks in Keil5 requires the following steps:
1. Double-click the "FreeRTOSConfig.h" file in the "Project" tab.
2. Configure FreeRTOS kernel parameters, such as the number of tasks, stack size, and clock speed.
3. Create tasks, specifying priority, stack size, and task functions for each task.
```c
/* FreeRTOSConfig.h */
#define configTOTAL_TASKS 3
#define configMINIMAL_STACK_SIZE 128
#define configCPU_CLOCK_HZ 8000000
/* Task 1 */
#define TASK1_PRIORITY 1
#define TASK1_STACK_SIZE 256
void Task1(void *pvParameters);
/* Task 2 */
#define TASK2_PRIORITY 2
#define TASK2_STACK_SIZE 256
void Task2(void *pvParameters);
/* Task 3 */
#define TASK3_PRIORITY 3
#define TASK3_STACK_SIZE 256
void Task3(void *pvParameters);
```
**Code Logic Analysis:**
* `configTOTAL_TASKS` defines the number of tasks in the system.
* `configMINIMAL_STACK_SIZE` defines the minimum stack size for each task.
* `configCPU_CLOCK_HZ` defines the CPU clock speed.
* `TASK1_PRIORITY`, `TASK2_PRIORITY`, and `TASK3_PRIORITY` define the priority for each task.
* `TASK1_STACK_SIZE`, `TASK2_STACK_SIZE`, and `TASK3_STACK_SIZE` define the stack size for each task.
* `Task1`, `Task2`, and `Task3` are task functions that will be scheduled for execution by FreeRTOS.
# 3. FreeRTOS Task Management
### 3.1 Task Creation and Control
#### 3.1.1 Task Creation Function and Parameters
In FreeRTOS, task creation uses the `xTaskCreate` function. The prototype of this function is as follows:
```cpp
BaseType_t xTaskCreate(
TaskFunction_t pvTaskCode, // Task function
```
0
0
相关推荐







