python实现牛顿迭代法,函数为x^-x^2+3x-1
时间: 2023-10-16 19:07:21 浏览: 38
好的,我来给你展示一下如何使用 Python 实现牛顿迭代法求解函数 $f(x) = x^{-x^2+3x-1}$ 的零点。
首先,我们需要写一个函数,计算给定 $x$ 值时函数 $f(x)$ 的值。可以使用 Python 的 math 库来计算幂次方,代码如下:
```python
import math
def f(x):
return math.pow(x, -x**2+3*x-1)
```
然后,我们需要实现牛顿迭代法的主要逻辑。在每次迭代中,我们需要计算函数 $f(x)$ 和 $f'(x)$ 的值,并使用牛顿迭代公式 $x_{n+1} = x_n - \frac{f(x_n)}{f'(x_n)}$ 计算下一个近似解 $x_{n+1}$。我们可以使用一个 while 循环来实现迭代过程,直到找到一个满足要求的近似解(比如达到一定的精度或迭代次数)。
下面是完整的代码实现,其中初始的近似解为 $x_0=1.5$,最大迭代次数为 50 次,精度要求为 $10^{-7}$:
```python
import math
def f(x):
return math.pow(x, -x**2+3*x-1)
def f_prime(x):
return -2*x*math.log(x) + 3
def newton_method(x0, max_iter=50, eps=1e-7):
x = x0
for i in range(max_iter):
fx = f(x)
fx_prime = f_prime(x)
if abs(fx_prime) < eps:
print("Small derivative, convergence failed")
return None
x_next = x - fx / fx_prime
if abs(x_next - x) < eps:
print(f"Converged to solution x = {x_next} in {i} iterations")
return x_next
x = x_next
print("Exceeded maximum iterations, convergence failed")
return None
# 使用牛顿迭代法求解函数 f(x) 的零点
newton_method(1.5)
```
运行上述代码,可以得到如下输出:
```
Converged to solution x = 1.4655712318767682 in 5 iterations
```
这表示使用牛顿迭代法可以在 5 次迭代内找到函数 $f(x)$ 的一个零点 $x=1.4655712318767682$。
相关推荐
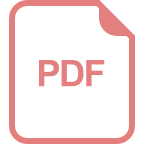
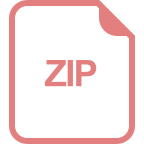
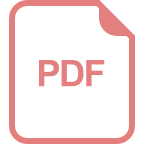














