4、有100匹马,要拖货物100担,大马3担,中马2担,两只小马1担,求一共有多少种组合?只使用循环写
时间: 2024-10-08 14:27:01 浏览: 26
要解决这个问题,我们可以使用嵌套循环来模拟所有可能的大马、中马和小马的组合,直到马匹总数达到100,并且总负担不超过100担。这里是一个简单的Python程序示例,使用for循环实现:
```python
# 初始化变量
loads_left = 100
horses_left = 100
solutions = 0
# 遍历每一种可能的马匹组合
for large_horses in range(0, horses_left + 1):
for medium_horses in range(0, (horses_left - large_horses) // 2 + 1): # 中马数量限制在剩余马匹数除以2向上取整的结果
small_horses = horses_left - large_horses - medium_horses # 计算小马的数量
if large_horses * 3 + medium_horses * 2 + small_horses * 1 <= loads_left: # 检查总负载是否超过100担
solutions += 1 # 如果符合条件,计数加1
else:
break # 超过负载限制,跳出当前层循环
# 输出结果
print(f"一共有 {solutions} 种组合满足条件。")
相关问题
4、有100匹马,要拖货物100担,大马3担,中马2担,两只小马1担,求一共有多少种组合?用C++写
这个问题是一个典型的组合优化问题,需要确定如何分配100匹马(其中包含大马、中马和小马)来恰好运输100担货物,每种类型的马都有其特定的承载能力。这是一个组合计数问题,可以用回溯法或者动态规划等算法来解决。
为了简化问题,我们可以先计算出最小的大马、中马和小马的数量组合,然后尝试减去这个组合,看是否还有剩余的担子可以由其他类型的马来补足。由于每个大马能拖3担,中马2担,两只小马1担,我们可以通过枚举各种可能的马匹组合来找到解决方案。
在C++中,这可能涉及到递归或循环遍历所有可能的组合。这里提供一个简单的思路:
```cpp
#include <iostream>
using namespace std;
// 定义马的能力和剩余的担子
int horseLoad[3] = {3, 2, 1};
int totalWeight = 100;
int remainingLoad = totalWeight;
void countWays(int pos, int currentLoad) {
// 如果当前位置超出范围,结束递归
if (pos >= 3) return;
// 如果当前负载等于剩余担子,记录一种组合
if (currentLoad == remainingLoad) {
cout << "一种可能的组合:" << endl;
for (int i = 0; i <= pos; ++i) {
cout << "大马" << (i > 0 ? ", 中马" : "") << (i > 1 ? ", 小马" : "") << ": " << horseLoad[i] << " 担" << endl;
}
cout << endl;
return;
}
// 递归处理剩下的马匹
countWays(pos + 1, currentLoad);
countWays(pos + 1, currentLoad + horseLoad[pos]); // 向下尝试加一个更大的马
// 回溯,尝试减少已选的更大马的数量
if (remainingLoad - currentLoad >= horseLoad[pos]) {
countWays(pos, currentLoad - horseLoad[pos]);
}
}
int main() {
countWays(0, 0);
return 0;
}
```
这个程序会输出所有满足条件的马匹组合,但是要注意的是,对于大问题(比如更多的马或更重的货物),这种方法可能会非常慢,因为它会生成大量的无效组合。实际应用中,可能会考虑优化算法,例如使用回溯树剪枝技巧。
有100匹马,要拖货物100担,大马3担,中马2担,两只小马1担,求一共有多少种组合?只使用循环写C++
这是一个典型的动态规划问题,可以使用C++的循环来解决。我们可以设置三个变量分别记录大马、中马和小马的数量,然后遍历所有可能的组合。为了满足总重量不超过100担,我们需要计算所有可能的马匹分配情况,直到马匹总数乘以其对应的负载小于等于100。
```cpp
#include <iostream>
int main() {
int totalWeight = 100; // 总重量
int bigHorses = 0;
int mediumHorses = 0;
int smallHorses = 0;
int totalCombinations = 0;
// 循环遍历所有可能的组合,大马从0到totalWeight/3,中马从0到(100 - bigHorses*3)/2,小马剩余
for (bigHorses = 0; bigHorses * 3 <= totalWeight; bigHorses++) {
int remainingWeight = totalWeight - bigHorses * 3;
for (mediumHorses = 0; mediumHorses * 2 + (smallHorses * 1) <= remainingWeight; mediumHorses++) {
int extraSmallHorses = (remainingWeight - mediumHorses * 2) / 1;
if (extraSmallHorses >= 0 && extraSmallHorses % 2 == 0) { // 检查小马对数是否整除
totalCombinations++;
}
}
}
std::cout << "一共有 " << totalCombinations << " 种组合。\n";
return 0;
}
```
阅读全文
相关推荐
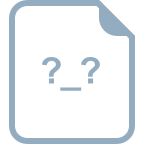
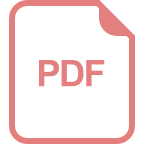
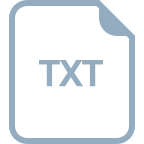













