【Foundation】Detailed Explanation of MATLAB Toolbox: Signal Processing Toolbox
发布时间: 2024-09-14 03:31:16 阅读量: 39 订阅数: 39 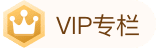
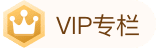
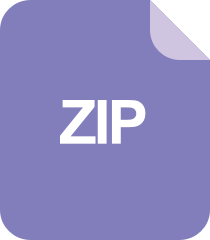
java+sql server项目之科帮网计算机配件报价系统源代码.zip
# 1. Introduction to the Signal Processing Toolbox
The Signal Processing Toolbox is a powerful toolset within MATLAB, designed for signal processing and analysis. It provides a comprehensive collection of functions and algorithms that empower engineers and researchers to process, analyze, and enhance signals effectively. From basic time-domain analysis to advanced array signal processing, the toolbox spans a wide array of signal processing tasks.
# 2.1 Representation and Analysis of Signals
### 2.1.1 Time-Domain and Frequency-Domain Analysis
**Time-Domain Analysis**
Time-domain analysis involves examining signals along the time axis. It reveals how the amplitude, phase, ***mon time-domain analysis methods include:
- **Oscilloscope:** Visualizes the time-domain waveform of signals.
- **Sampling Theorem:** Dictates that a signal's sampling frequency must be at least twice the highest frequency to reconstruct the signal without distortion.
- **Nyquist Frequency:** Half the highest frequency of the signal, which is the upper limit for the sampling frequency.
**Frequency-Domain Analysis**
Frequency-domain analysis involves examining signals along the frequen***mon frequency-domain analysis methods include:
- **Fourier Transform:** Converts time-domain signals into the frequency domain, revealing the amplitude and phase of different frequency components.
- **Discrete Fourier Transform (DFT):** The discrete version of the Fourier Transform, used for analyzing discrete-time signals.
- **Fast Fourier Transform (FFT):** A fast algorithm for DFT that significantly improves computational efficiency.
### 2.1.2 Fourier Transform and Discrete Fourier Transform
**Fourier Transform**
The Fourier Transform is a mathematical transformation that converts a time-domain signal into a frequency-domain signal. It uncovers the amplitude and phase of the signal's different frequency components. The Fourier Transform formula is:
```
X(f) = ∫_{-∞}^{∞} x(t) e^(-j2πft) dt
```
Where:
- `X(f)` is the frequency-domain signal
- `x(t)` is the time-domain signal
- `f` is the frequency
- `j` is the imaginary unit
**Discrete Fourier Transform (DFT)**
DFT is the discrete version of the Fourier Transform, used to analyze discrete-time signals. The DFT formula is:
```
X[k] = ∑_{n=0}^{N-1} x[n] e^(-j2πkn/N)
```
Where:
- `X[k]` is the k-th sample value of the frequency-domain signal
- `x[n]` is the n-th sample value of the time-domain signal
- `N` is the length of the signal
- `k` is the frequency index
# 3. Signal Processing Toolbox in Practice
### 3.1 Signal Analysis
#### 3.1.1 Spectral Analysis
Spectral analysis is a fundamental task in signal processing, used to study the frequency components of a signal. The Signal Processing Toolbox provides various functions for spectral analysis, including:
- `fft`: Computes the Discrete Fourier Transform (DFT)
- `spectrogram`: Computes the Short-Time Fourier Transform (STFT)
- `pwelch`: Computes the Power Spectral Density (PSD)
**Code Block: Computing the Spectrum of a Signal**
```matlab
% Generate a sine signal
t = 0:0.01:1;
f = 10;
x = sin(2 * pi * f * t);
% Compute DFT
X = fft(x);
% Compute magnitude spectrum
magnitude = abs(X);
% Plot magnitude spectrum
figure;
stem(magnitude);
xlabel('Frequency (Hz)');
ylabel('Magnitude');
title('DFT of the Signal');
```
**Logical Analysis:**
* The `fft` function computes the DFT of the signal, storing the result in `X`.
* The `abs` function calculates the magnitude of the complex `X`, resulting in the magnitude spectrum.
* The `stem` function plots the magnitude spectrum, displaying the frequency components of the signal.
#### 3.1.2 Correlation and Coherence Analysis
Correlation and coherence analysis are used to measure the similarity and relationship between two signals. The Signal Processing Toolbox offers the following functions:
- `corrcoef`: Computes the correlation coefficient
- `xcorr`: Computes the cross-correlation
- `mscohere`: Computes the coherence
**Code Block: Computing the Correlation of Two Signals**
```matlab
% Generate two sine signals
t = 0:0.01:1;
f1 = 10;
f2 = 12;
x1 = sin(2 * pi * f1 * t);
x2 = sin(2 * pi * f2 * t);
% Compute correlation coefficient
corr = corrcoef(x1, x2);
% Print correlation coefficient
disp(['Correlation coefficient: ', num2str(corr(1, 2))]);
```
**Logical Analysis:**
* The `corrcoef` function calculates the correlation coefficient between two signals `x1` and `x2`, storing the result in `corr`.
* The `disp` function prints the correlation coefficient, indicating the correlation between the signals.
### 3.2 Filtering
#### 3.2.1 Digital Filter Implementation
The Signal Processing Toolbox provides various functions to implement digital filters, including:
- `filter`: Applies filter to signal
- `designfilt`: Designs filters
- `freqz`: Analyzes the frequency response of filters
**Code Block: Designing and Applying a Low-Pass Filter**
```matlab
% Design a low-pass filter
order = 10;
cutoff_freq = 0.2;
[b, a] = butter(order, cutoff_freq);
% Apply the filter
y = filter(b, a, x);
% Plot the filtered signal
figure;
plot(t, y);
xlabel('Time (s)');
ylabel('Amplitude');
title('Filtered Signal');
```
**Logical Analysis:**
* The `butter` function designs a low-pass filter with the specified `order` and `cutoff_freq`, returning filter coefficients `b` and `a`.
* The `filter` function applies the filter to the signal `x`, resulting in the filtered signal `y`.
* The `plot` function graphs the filtered signal, displaying the filtering effect.
#### 3.2.2 Filter Design and Optimization
The Signal Processing Toolbox offers various methods for designing and optimizing filters, including:
- `fdatool`: Graphical filter design tool
- `fdesign`: Filter design object
- `optimset`: Optimization options
**Code Block: Using `fdatool` to Design a Filter**
```matlab
% Open the graphical filter design tool
fdatool;
% Design a low-pass filter
order = 10;
cutoff_freq = 0.2;
h = fdesign.lowpass('N,F3dB', order, cutoff_freq);
% Apply the filter
y = filter(h, x);
% Plot the filtered signal
figure;
plot(t, y);
xlabel('Time (s)');
ylabel('Amplitude');
title('Filtered Signal');
```
**Logical Analysis:**
* The `fdatool` function opens the graphical filter design tool, allowing users to design filters interactively.
* The `fdesign.lowpass` function creates a low-pass filter design object `h`, specifying the filter order and cutoff frequency.
* The `filter` function applies filter `h` to the signal `x`, resulting in the filtered signal `y`.
* The `plot` function graphs the filtered signal, displaying the filtering effect.
# 4. Advanced Signal Processing Techniques
### 4.1 Waveform Analysis
#### 4.1.1 Time-Frequency Analysis
**Time-Frequency Analysis** is a technique that represents a signal in both the time and frequency domains simultaneously. It is realized through the use of time-frequency distributions (TFD), which are two-dimensional functions. The horizontal axis represents time, the vertical axis represents frequency, and the values represent the signal's energy at specific times and frequencies.
**Types of Time-Frequency Distributions**
***Short-Time Fourier Transform (STFT):** Divides the signal into overlapping frames and performs a Fourier transform on each frame.
***Wavelet Transform (WT):** Uses a set of functions called wavelets for multiscale analysis of the signal.
***Hilbert-Huang Transform (HHT):** Decomposes the signal into oscillatory components called intrinsic mode functions (IMF).
**Applications**
* Fault diagnosis
* Speech recognition
* Music analysis
#### 4.1.2 Wavelet Transform
**Wavelet Transform** is a time-frequency analysis technique that uses a set of functions called wavelets for multiscale analysis of signals. Wavelets are localized functions with finite duration and oscillation.
**Advantages of Wavelet Transform**
***Multiscale Analysis:** Wavelet transform can analyze signals at different scales, allowing it to capture features within various frequency ranges.
***Time-Frequency Localization:** Wavelet transform can locate signal features in both time and frequency domains simultaneously.
***Robustness:** Wavelet transform is robust against noise and non-stationary signals.
**Applications**
* Image processing
* Signal denoising
* Feature extraction
### 4.2 Adaptive Signal Processing
#### 4.2.1 Adaptive Filtering
**Adaptive Filtering** is a type of filter that can automatically adjust its filter coefficients to adapt to changes in the signal. It updates filter coefficients using an error signal, thus achieving adaptability.
**Adaptive Filtering Algorithms**
***Least Mean Squares (LMS):** Uses mean squared error as the adaptive criterion.
***Recursive Least Squares (RLS):** Uses recursive least squares estimation as the adaptive criterion.
***Kalman Filter:** Uses the Kalman filter as the adaptive criterion.
**Applications**
* Noise reduction
* System identification
* Echo cancellation
#### 4.2.2 Adaptive Noise Cancellation
**Adaptive Noise Cancellation** is a technique that uses adaptive filtering to remove noise. It is achieved by estimating the noise signal and subtracting it from the main signal.
**Adaptive Noise Cancellation Algorithms**
***Frequency Domain Adaptive Filtering (FDAF):** Implements adaptive filtering in the frequency domain.
***Time Domain Adaptive Filtering (TDAF):** Implements adaptive filtering in the time domain.
***Blind Source Separation (BSS):** Separates multiple source signals from a mixed signal.
**Applications**
* Speech enhancement
* Image denoising
* Biomedical signal processing
### 4.3 Array Signal Processing
#### 4.3.1 Beamforming
**Beamforming** is a technique that uses multiple sensor arrays to enhance signals from a specific direction. It is realized by adjusting the phase of each sensor's signal in the array, forming a beam in a particular direction.
**Beamforming Algorithms**
***Delay-and-Sum Beamforming (DSBF):** A simple yet effective beamforming algorithm.
***Adaptive Beamforming (ABF):** An adaptive beamforming algorithm that can adapt to signal changes.
***Minimum Variance Distortionless Response (MVDR):** A beamforming algorithm that produces a beam with minimum variance and undistorted response.
**Applications**
* Radar
* Sonar
* Communications
#### 4.3.2 Direction of Arrival Estimation
**Direction of Arrival Estimation** is a technique for determining the direction of signal sources. It uses array signal processing techniques, such as beamforming, to estimate the Angle of Arrival (AOA) of the signal.
**Direction of Arrival Estimation Algorithms**
***MUSIC (MUltiple SIgnal Classification):** A subspace decomposition-based direction of arrival estimation algorithm.
***ESPRIT (Estimation of Signal Parameters via Rotational Invariance Techniques):** Another subspace decomposition-based direction of arrival estimation algorithm.
***MLE (Maximum Likelihood Estimation):** A direction of arrival estimation algorithm based on the maximum likelihood criterion.
**Applications**
* Radar
* Sonar
* Wireless communication
# 5. Signal Processing Toolbox Applications
The Signal Processing Toolbox is widely used in various fields, including speech signal processing, image processing, and biomedical signal processing. This chapter will introduce typical applications in these fields and demonstrate how to use the Signal Processing Toolbox to solve real-world problems.
### 5.1 Speech Signal Processing
Speech signal processing involves the analysis, processing, and synthesis of speech signals. The Signal Processing Toolbox provides a range of tools for speech recognition, speech synthesis, speech enhancement, and speech coding.
**5.1.1 Speech Recognition**
Speech recognition systems convert speech signals into text. The Signal Processing Toolbox provides functions for feature extraction, model training, and recognition algorithms.
```
% Import speech signal
speechSignal = audioread('speech.wav');
% Preprocess the speech signal
preprocessedSignal = preprocess(speechSignal);
% Feature extraction
features = extractFeatures(preprocessedSignal);
% Train the speech recognition model
model = trainModel(features, labels);
% Recognize speech
recognizedText = recognizeSpeech(model, speechSignal);
```
**5.1.2 Speech Synthesis**
Speech synthesis systems convert text into speech. The Signal Processing Toolbox provides functions for text-to-speech conversion, speech quality assessment, and speech synthesis algorithms.
```
% Import text
text = 'Hello, world!';
% Text-to-speech conversion
syntheticSpeech = text2speech(text);
% Play the synthesized speech
sound(syntheticSpeech);
```
### 5.2 Image Processing
Image processing involves the analysis, processing, and enhancement of image data. The Signal Processing Toolbox provides a range of tools for image enhancement, image segmentation, image compression, and image analysis.
**5.2.1 Image Enhancement**
Image enhancement techniques are used to improve the visual quality of images. The Signal Processing Toolbox provides functions for contrast enhancement, sharpening, noise reduction, and color correction.
```
% Import image
image = imread('image.jpg');
% Contrast enhancement
enhancedImage = imadjust(image, [0.2 0.8], []);
% Sharpening
sharpenedImage = imsharpen(image, 'Radius', 2, 'Amount', 1);
% Denoising
denoisedImage = wiener2(image, [5 5]);
```
**5.2.2 Image Segmentation**
Image segmentation divides an image into different regions or objects. The Signal Processing Toolbox provides functions for edge detection, region growing, and clustering.
```
% Import image
image = imread('image.jpg');
% Edge detection
edges = edge(image, 'canny');
% Region growing
segmentedImage = regiongrow(image, [***]);
% Clustering
clusters = kmeans(image(:), 3);
segmentedImage = reshape(clusters, size(image));
```
### 5.3 Biomedical Signal Processing
Biomedical signal processing involves the analysis, processing, and interpretation of biomedical signals. The Signal Processing Toolbox provides a range of tools for electrocardiogram analysis, electroencephalogram analysis, and medical image processing.
**5.3.1 Electrocardiogram Analysis**
Electrocardiogram (ECG) analysis is used for diagnosing heart diseases. The Signal Processing Toolbox provides functions for ECG signal preprocessing, feature extraction, and arrhythmia detection.
```
% Import ECG signal
ecgSignal = load('ecg.mat').ecg;
% Preprocess ECG signal
preprocessedSignal = preprocessEcg(ecgSignal);
% Feature extraction
features = extractFeaturesEcg(preprocessedSignal);
% Arrhythmia detection
arrhythmias = detectArrhythmias(features);
```
**5.3.2 Electroencephalogram Analysis**
Electroencephalogram (EEG) analysis is used for diagnosing neurological diseases. The Signal Processing Toolbox provides functions for EEG signal preprocessing, feature extraction, and brain activity analysis.
```
% Import EEG signal
eegSignal = load('eeg.mat').eeg;
% Preprocess EEG signal
preprocessedSignal = preprocessEeg(eegSignal);
% Feature extraction
features = extractFeaturesEeg(preprocessedSignal);
% Brain activity analysis
brainActivity = analyzeBrainActivity(features);
```
# 6. Signal Processing Toolbox Extensions
### 6.1 Integration with Other Toolboxes
The Signal Processing Toolbox can be integrated with other toolboxes in MATLAB to extend its capabilities and solve more complex problems.
#### 6.1.1 Integration with Simulink
Simulink is a graphical environment for modeling, simulating, and analyzing dynamic systems. The integration of the Signal Processing Toolbox with Simulink allows users to embed signal processing algorithms directly into Simulink models. This enables real-time signal processing and analysis and the design and simulation of complex signal processing systems.
#### 6.1.2 Integration with Statistics and Machine Learning Toolbox
The Statistics and Machine Learning Toolbox provides functions for statistical analysis, machine learning, and data mining. The integration of the Signal Processing Toolbox with the Statistics and Machine Learning Toolbox allows users to combine signal processing techniques with statistical and machine learning methods. This facilitates advanced signal analysis tasks such as signal classification, dimensionality reduction, and anomaly detection.
### 6.2 Third-Party Extensions and Community Resources
In addition to the built-in extensions in MATLAB, there are many third-party extensions and community resources available to enhance the functionality of the Signal Processing Toolbox.
#### 6.2.1 Proprietary Toolboxes and Function Libraries
Many third-party developers have created proprietary toolboxes and function libraries to extend the functionality of the Signal Processing Toolbox. These extensions can provide additional algorithms, optimization techniques, and tools for specific domains.
#### 6.2.2 Online Forums and Discussion Groups
The MATLAB community has active online forums and discussion groups where users can share knowledge, seek help, and discuss the use of the Signal Processing Toolbox. These resources are invaluable for obtaining information on extensions, best practices, and troubleshooting.
0
0
相关推荐
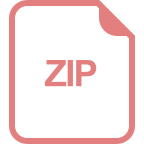
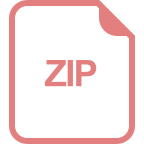
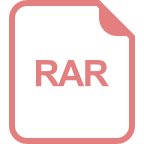
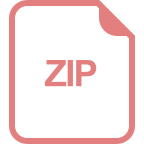
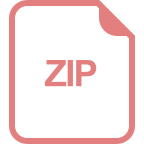