【Advanced】Single-Phase Half-Wave Controlled Rectifier Circuit Simulink MATLAB Simulation Model
发布时间: 2024-09-14 04:26:27 阅读量: 42 订阅数: 45 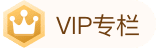
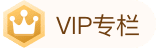
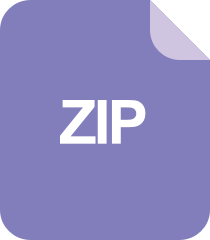
single_phase_half_controlled rectifier:single_phase_half_controlled rectifier using simulink-matlab开发
# 1. Modeling Circuit Components
Modeling of circuit components is crucial in Simulink as it determines the accuracy and reliability of the simulation model. Simulink offers a rich library of pre-defined models for a variety of circuit components.
**2.1.1 Diode Model**
The diode model in Simulink is a module used to simulate the behavior of diodes. It has two terminals, an anode, and a cathode. When the anode voltage is higher than the cathode voltage, the diode conducts, allowing current to flow from the anode to the cathode. Otherwise, the diode is off, ***mon diode models in Simulink include the ideal diode, Schottky diode, and Zener diode.
**Code Block:**
```
% Create an ideal diode
ideal_diode = ***ponents.IdealDiode;
% Set diode parameters
ideal_diode.ForwardVoltageDrop = 0.7; % Forward voltage drop
ideal_diode.ReverseBreakdownVoltage = -100; % Reverse breakdown voltage
```
# 2. Simulink Modeling Techniques
Simulink is a powerful tool in MATLAB for modeling and simulating dynamic systems. In the simulation of a single-phase half-wave controlled rectifier circuit, Simulink provides an intuitive graphical interface that makes it easy to construct and simulate circuit models. This section will introduce Simulink modeling techniques, including the modeling of circuit components and the setup of simulation parameters.
### 2.1 Modeling of Circuit Components
Simulink provides a series of libraries with pre-built models of various circuit components. These models can be conveniently dragged into Simulink models to quickly build circuit models.
#### 2.1.1 Diode Model
The diode is a key component in a single-phase half-wave controlled rectifier circuit. Simulink offers various diode models, including the ideal diode, Schottky diode, and Zener diode. For the single-phase half-wave controlled rectifier circuit, the ideal diode model is commonly used.
```
% Create an ideal diode model
diode = ***ponents.IdealDiode;
% Set diode parameters
diode.ForwardVoltageDrop = 0.7; % Forward voltage drop
diode.ReverseBreakdownVoltage = -100; % Reverse breakdown voltage
```
#### 2.1.2 Inductor Model
Inductors are also important components in a single-phase half-wave controlled rectifier circuit. Simulink offers various inductor models, including the ideal inductor, saturable inductor, and transformer. For the single-phase half-wave controlled rectifier circuit, the ideal inductor model is commonly used.
```
% Create an ideal inductor model
inductor = ***ponents.Inductor;
% Set inductor parameters
inductor.Inductance = 10e-3; % Inductance value
```
#### 2.1.3 Resistor Model
Resistors are commonly used components in a single-phase half-wave controlled rectifier circuit. Simulink offers various resistor models, including the ideal resistor, nonlinear resistor, and thermistor. For the single-phase half-wave controlled rectifier circuit, the ideal resistor model is commonly used.
```
% Create an ideal resistor model
resistor = ***ponents.Resistor;
% Set resistor parameters
resistor.Resistance = 100; % Resistance value
```
### 2.2 Setting Simulation Parameters
Simulation parameters are important factors that affect the results of Simulink simulations. These parameters include sample time, simulation step size, and simulation time.
#### 2.2.1 Sample Time
Sample time is the interval used by Simulink to discretize continuous-time models. A smaller sample time can improve simulation accuracy but will also increase simulation time. For the single-phase half-wave controlled rectifier circuit, a sample time of 1e-6 seconds is commonly used.
#### 2.2.2 Simulation Step Size
Simulation step size is the interval Simulink uses to solve differential equations. A smaller step size can improve simulation accuracy but will also increase simulation time. For the single-phase half-wave controlled rectifier circuit, a simulation step size of 1e-6 seconds is commonly used.
#### 2.2.3 Simulation Time
Simulation time is the total time Simulink uses to run simulations. The simulation time should be long enough to capture both the steady-state and transient responses of the circuit. For the single-phase half-wave controlled rectifier circuit, a simulation time of 0.1 seconds is commonly used.
# 3. MATLAB Simulation Programming
### 3.1 Signal Generation
MATLAB provides a rich library of functions to easily generate various types of signals.
#### 3.1.1 Sine Signal
```
t = 0:0.001:1; % Time vector
f = 50; % Frequency
A = 1; % Amplitude
x = A * sin(2 * pi * f * t); % Sine signal
```
**Code Logic Interpretation:**
* `t` is a time vector that specifies the sampling time interval of the signal.
* `f` is the frequency of the sine signal.
* `A` is the amplitude of the sine signal.
* `x` is the generated sine signal.
#### 3.1.2 Pulse Signal
```
t = 0:0.001:1; % Time vector
f = 50; % Frequency
A = 1; % Amplitude
duty = 0.5; % Duty cycle
x = A * square(2 * pi * f * t, duty); % Pulse signal
```
**Code Logic Interpretation:**
* `t` is a time vector that specifies the sampling time interval of the signal.
* `f` is the frequency of the pulse signal.
* `A` is the amplitude of the pulse signal.
* `duty` is the duty cycle of the pulse signal.
* `x` is the generated pulse signal.
#### 3.1.3 Random Signal
```
t = 0:0.001:1; % Time vector
n = randn(size(t)); % Generate normally distributed random numbers
x = n; % Random signal
```
**Code Logic Interpretation:**
* `t` is a time vector that specifies the sampling time interval of the signal.
* `n` is a normally distributed random number.
* `x` is the generated random signal.
### 3.2 Data Processing
MATLAB provides powerful data processing capabilities that can perform various operations on signals.
#### 3.2.1 Signal Filtering
```
x = randn(1000); % Generate normally distributed random signal
y = filter(b, a, x); % Filtered signal
```
**Code Logic Interpretation:**
* `x` is a normally distributed random signal.
* `b` and `a` are filter coefficients.
* `y` is the filtered signal.
#### 3.2.2 Data Visualization
```
t = 0:0.001:1; % Time vector
x = sin(2 * pi * 50 * t); % Sine signal
plot(t, x); % Plot the sine signal
```
**Code Logic Interpretation:**
* `t` is a time vector that specifies the sampling time interval of the signal.
* `x` is a sine signal.
* `plot(t, x)` plots the sine signal.
### 3.3 Result Analysis
MATLAB provides a rich set of analysis tools that can perform various analyses on signals.
#### 3.3.1 Waveform Analysis
```
t = 0:0.001:1; % Time vector
x = sin(2 * pi * 50 * t); % Sine signal
[peak, peak_index] = max(x); % Find peak value
[trough, trough_index] = min(x); % Find trough value
```
**Code Logic Interpretation:**
* `t` is a time vector that specifies the sampling time interval of the signal.
* `x` is a sine signal.
* `max(x)` finds the peak value of the sine signal.
* `min(x)` finds the trough value of the sine signal.
#### 3.3.2 Harmoni
0
0
相关推荐





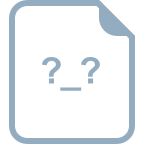

