Introduction to MATLAB GUI Programming Essentials
发布时间: 2024-09-14 04:06:32 阅读量: 34 订阅数: 47 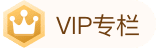
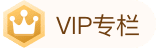
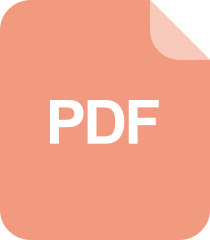
Introduction to Python Programming and developing GUI applications with PyQT
# 1. Basic Concepts of MATLAB GUI Programming
MATLAB Graphical User Interface (GUI) programming is a method to create interactive interfaces that allow users to interact with MATLAB programs. A MATLAB GUI consists of various controls, such as buttons, text boxes, and checkboxes, organized within layouts like panels, flow layouts, and grid layouts. Event handling functions, such as anonymous functions and callback functions, respond to user interactions with these controls. MATLAB GUI programming offers an intuitive way to create user-friendly applications, simplifying interactions with MATLAB programs.
# 2.1 Basic Controls
### 2.1.1 Buttons
Buttons are the most common controls in a GUI, used to trigger events. Button controls in MATLAB can be created using the `uicontrol` function, with the syntax as follows:
```
btn = uicontrol('Style', 'pushbutton', ...
'String', 'Button Text', ...
'Position', [x1, y1, width, height], ...
'Callback', @callback_function);
```
**Parameter Explanation:**
* `Style`: Specifies the type of the control, in this case, `pushbutton`.
* `String`: The text on the button.
* `Position`: The location and size of the button, specified in the form [x1, y1, width, height].
* `Callback`: The callback function triggered when the button is clicked.
**Code Logic Analysis:**
This code creates a button control and specifies its text, location, and callback function. When the user clicks the button, the `callback_function` is called to handle the event.
### 2.1.2 Text Boxes
Text boxes are used for input and display of text. Text box controls in MATLAB can be created using the `uicontrol` function, with the syntax as follows:
```
txt = uicontrol('Style', 'edit', ...
'String', 'Initial Text', ...
'Position', [x1, y1, width, height]);
```
**Parameter Explanation:**
* `Style`: Specifies the type of the control, in this case, `edit`.
* `String`: The initial text in the text box.
* `Position`: The location and size of the text box, specified in the form [x1, y1, width, height].
**Code Logic Analysis:**
This code creates a text box control and specifies its initial text and location. Users can input or edit text in the text box.
### 2.1.3 Checkboxes
Checkboxes are used to represent a binary state (checked or unchecked). Checkbox controls in MATLAB can be created using the `uicontrol` function, with the syntax as follows:
```
chk = uicontrol('Style', 'checkbox', ...
'String', 'Checkbox Text', ...
'Position', [x1, y1, width, height], ...
'Value', 0);
```
**Parameter Explanation:**
* `Style`: Specifies the type of the control, in this case, `checkbox`.
* `String`: The text next to the checkbox.
* `Position`: The location and size of the checkbox, specified in the form [x1, y1, width, height].
* `Value`: The initial state of the checkbox, 0 indicates unchecked, 1 indicates checked.
**Code Logic Analysis:**
This code creates a checkbox control and specifies its text, location, and initial state. Users can toggle the state by clicking the checkbox.
# 3. MATLAB GUI Programming Event Handling
### 3.1 Event Types
In MATLAB GUI programming, events are actions t
0
0
相关推荐







