【Advanced】PID Controller MATLAB Simulation
发布时间: 2024-09-14 04:35:09 阅读量: 14 订阅数: 22 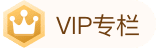
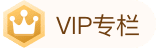
# Theoretical Foundation of PID Controllers
PID (Proportional-Integral-Derivative) controllers are widely used in industrial automation and robotic control as feedback control algorithms. The fundamental principle involves measuring the deviation between the output signal of the controlled object and the desired value, and generating corresponding control signals based on the magnitude and rate of change of this deviation, to bring the output signal of the controlled object as close as possible to the desired value.
The mathematical model of a PID controller consists of three components: the proportional term, the integral term, and the derivative term. The proportional term is proportional to the deviation, the integral term is proportional to the integral of the deviation, and the derivative term is proportional to the rate of change of the deviation. By adjusting the coefficients of these three terms, the response characteristics of the controller can be altered to meet different control requirements.
# 2. Simulation of PID Controllers in MATLAB
### 2.1 Modeling a PID Controller in MATLAB
#### 2.1.1 Continuous-Time Model
In the continuous time domain, the mathematical model of a PID controller can be represented as:
```matlab
u(t) = Kp * e(t) + Ki * ∫e(t)dt + Kd * de(t)/dt
```
Where:
- `u(t)` is the control signal,
- `e(t)` is the error signal (the difference between the reference value and the actual value),
- `Kp` is the proportional gain,
- `Ki` is the integral gain,
- `Kd` is the derivative gain.
In MATLAB, the `tf` function can be used to create a continuous-time transfer function model:
```matlab
num = [Kp, Ki, Kd];
den = [1, 0, 0];
PID_continuous = tf(num, den);
```
#### 2.1.2 Discrete-Time Model
For practical control systems, it is often necessary to convert a continuous-time model into a discrete-time model. In MATLAB, this can be achieved using the `c2d` function:
```matlab
Ts = 0.1; % Sampling time
PID_discrete = c2d(PID_continuous, Ts);
```
The mathematical model for a discrete-time PID controller is:
```matlab
u(k) = Kp * e(k) + Ki * Ts * ∑e(i) + Kd * (e(k) - e(k-1)) / Ts
```
Where:
- `k` is the discrete-time step.
### 2.2 Methods for Tuning PID Parameters
#### 2.2.1 Ziegler-Nichols Method
The Ziegler-Nichols method is a classic approach for tuning PID parameters, which determines the parameters by observing the system's step response curve. The steps are as follows:
1. Switch the PID controller to pure proportional control (`Ki` and `Kd` set to 0).
2. Gradually increase `Kp` until the system exhibits sustained oscillations.
3. Record the oscillation period `T` and amplitude `A`.
4. Calculate the PID parameters based on `T` and `A`:
```
Kp = 0.6 * Kcu
Ki = 2 * Kcu / T
Kd = Kcu * T / 8
```
Where `Kcu` is the ultimate gain (the value of `Kp` when the system exhibits sustained oscillations).
#### 2.2.2 Cohen-Coon Method
The Cohen-Coon method is another frequently used method for tuning PID parameters, which is based on the system's process time constant (`τ`) and dead time (`L`). The steps are as follows:
1. Determine the system's process time constant and dead time.
2. Calculate the PID parameters based on `τ` and `L`:
```
Kp = 1.2 * (1 + L / 2 / τ) / Kp
Ki = 0.6 * Kp / τ
Kd = 0.6 * Kp * L / τ
```
# 3. PID Controller Simulation Experiments
### 3.1 System Identification and Model Building
Simulation experiments for PID controllers require the establishment of a mathematical model of the controlled system. Sy***mon system identification methods include:
- **Step Response Method:** Apply a step input to the system, record the output response, and fit the system's mathematical model based on the output response.
- **Impulse Response Method:** Apply an impulse input to the system, record the output response, and fit the system's mathematical model based on the output response.
- **Frequency Response Method:** Apply a sinusoidal input to the system, record the output response, and fit the system's mathematical model based on the output response.
In MATLAB, the `ident` toolbox can be used for system identification. The `ident` toolbox offers a variety of system identification methods, such as:
```matlab
% Step Response Method
data = iddata(y, u, Ts);
sys = tfest(data, 2);
% Impulse Response Method
data = iddata(y, u, Ts);
sys = impulseest(data, 2);
% Frequency Response Method
data = iddata(y, u, Ts);
sys = freqest(data, 2);
```
Wher
0
0
相关推荐
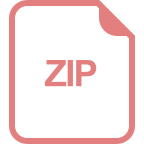
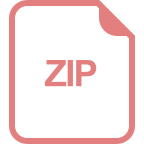
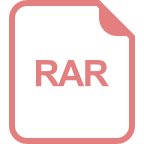
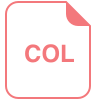
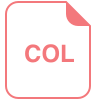
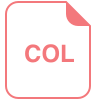
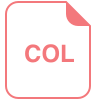

