[Advanced] Simulink Simulation of Progressive Stability of Neural Networks
发布时间: 2024-09-14 04:11:33 阅读量: 39 订阅数: 39 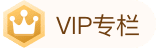
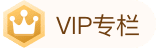
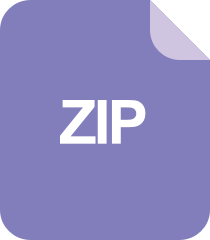
COAT_everywhere6of_Simulation_
# 2.1 Definition and Properties of Asymptotic Stability
Asymptotic stability is a key concept in measuring system stability in neural networks. It describes whether a system can recover to its equilibrium point or desired state after being perturbed. The definition of asymptotic stability is as follows:
For a given neural network system, if there exists a positive number ε>0, such that for any initial state x(0)∈R^n, as long as it satisfies:
```
||x(0) - x*|| < ε
```
Then for all t≥0, it holds that:
```
||x(t) - x*|| < ε
```
where x(t) is the state of the neural network at time t, and x* is the equilibrium point of the neural network.
Asymptotic stability has the following properties:
***Locality:** Asymptotic stability only guarantees that the system is stable near the equilibrium point.
***Attractiveness:** An asymptotically stable system will attract all states in its neighborhood.
***Consistency:** An asymptotically stable system is stable for all initial states.
# 2. Neural Network Asymptotic Stability Theory
### 2.1 Definition and Properties of Asymptotic Stability
Asymptotic stability is an important concept in neural network theory, describing the ability of neural networks to converge to an equilibrium point or trajectory after being disturbed. The definition of asymptotic stability is as follows:
For a neural network system, if there exists a positive number ε>0, such that for any initial state x(0)∈R^n, when ||x(0)-x*||<ε, the system state x(t) satisfies:
```
lim_{t->∞} ||x(t)-x*|| = 0
```
where x* is the equilibrium point or trajectory of the neural network.
Asymptotic stability has the following properties:
***Basin of Attraction:** An asymptotically stable neural network system has a basin of attraction, which is a set that contains all initial states such that the system state starting from this set can converge to the equilibrium point or trajectory.
***Convergence Rate:** The convergence rate of asymptotic stability depends on system parameters and the initial state.
***Robustness:** An asymptotically stable neural network system is robust against disturbances, meaning that the system can still remain stable within a certain range of disturbances.
### 2.2 Methods for Analyzing Asymptotic Stability
The main methods for analyzing neural network asymptotic stability include:
#### 2.2.1 Lyapunov Stability Theory
Lyapunov stability theory is a classical method for analyzing neural network asymptotic stability. This theory is based on a Lyapunov function, which is a non-negative function defined on the state space of the neural network, satisfying the following conditions:
* V(x)>0, for all x≠x*
* V(x*)=0
* For all x≠x*, there exists a continuous function W(x) such that:
```
dV(x)/dt ≤ -W(x)
```
If such a Lyapunov function exists, then the neural network system is asymptotically stable.
#### 2.2.2 Input-Output Stability Theory
Input-output stability theory is another method for analyzing neural network asymptotic stability. This theory is based on the input-output relationship, which is the relationship between the output y(t) and input u(t) of the neural network. The definition of input-output stability theory is as follows:
For a neural network system, if there exists a function V(y) such that for all inputs u(t) and initial states x(0), the system output y(t) satisfies:
```
lim_{t->∞} V(y(t)) = 0
```
Then the neural network system is asymptotically stable.
### 2.3 Design Criteria for Asymptotic Stability
The main design criteria for neural network asymptotic stability include:
#### 2.3.1 Linear Matrix Inequality (LMI) Method
The LMI method is a common method for designing neural network asymptotic stability. This method is based on linear matrix inequalities, which have the following form:
```
F(x) + G(x)Y+Y^T G^T(x) < 0
```
where F(x) and G(x) are matrices related to the state x of the neural network, and Y is a待定 matrix. If there exists a Y that satisfies this inequality, then the neural network system is asymptotically stable.
#### 2.3.2 Matrix Inequality (MI) Method
The MI method is another method for designing neural network asymptotic stability. This method is based on matrix inequalities, which have the following form:
```
A + XB + B^T X^T < 0
```
where A and B are matrices related to the state x of the neural network, and X is a待定 matrix. If there exists an X that satisfies this inequality, then the neural network system is asymptotically stable.
# 3. Simulink Simulation Practice of Neural Network Asymptotic Stability
### 3.1 Introduction to the Simulink Simulation Platform
Simulink is a graphical simulation environment developed by MathWorks, widely used for modeling, simulating, and analyzing dynamic systems. It offers a rich set of libraries and toolboxes, including a neural network model library, making it convenient to build and simulate neural network systems.
### 3.2 Simulink Implementation of Neural Network Models
**Code Block 1: Simulink Implementation of Neural Network Models**
```
% Create neural network model
net = feedforwardnet([10 10 1]);
net = train(net, inputData, targetData);
% Build Simulink model
simulinkModel = new_system('NeuralNetworkModel');
add_block('nnet/Neural Network', [simulinkModel '/Neural Network']);
set_param([simulinkModel '/Neural Network'], 'Network', net);
```
**Logical Analysis:**
* The `feedforwardnet` function creates a three-layer feedforward neural network with 10 hidden layer neurons and 10 output layer neurons.
* The `train` function trains the neural network using the input data `inputData` and target data `targetData`.
* The `new_system` function creates a new Simulink model.
* The `add_block` function adds a neural network module to the Simulink model.
* The `set_param` function sets the parameters of the neural network module, including the trained neural network `net`.
### 3.3 Simulink Implementation of Asymptotic Stability Analysis
#### 3.3.1 Lyapunov Function Method
**Code Block 2: Simulink Implementation of Lyapunov Function Method for Asymptotic Stability Analysis**
```
% Define Lyapunov function
V = @(x) x'*P*x;
% Build Simulink model
simulinkModel = new_system('LyapunovStabilityAnalysis');
add_block('simulink/Sources/Constant', [simulinkModel '/Initial Condition']);
set_param([simulinkModel '/Initial Condition'], 'Value', '1');
add_block('simulink/Continuous/State-Space', [simulinkModel '/Neural Network']);
set_param([simulinkModel '/Neural Network'], 'A', A);
set_param([simulinkModel '/Neural Network'], 'B', B);
add_block('simulink/Sinks/Out1', [simulinkModel '/Output']);
add_block('simulink/Math Operations/Dot Product', [simulinkModel '/Lyapunov Function']);
set_param([simulinkModel '/Lyapunov Function'], 'Inputs', '[1, 2]');
add_block('simulink/Sinks/Out1', [simulinkModel '/Lyapunov Value']);
```
**Logical Analysis:**
* The `V` function defines the Lyapunov function.
* The `new_system` function creates a new Simulink model.
* The `add_block` function adds modules to the Simulink model.
* The `set_param` function sets the parameters of the modules, including the state space matrices `A` and `B`.
* The `Dot Product` module calculates the value of the Lyapunov function.
#### 3.3.2 Input-Output Stability Method
**Code Block 3: Sim
0
0
相关推荐
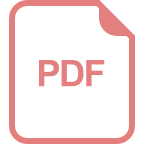
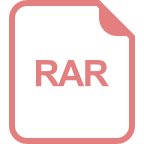
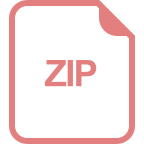
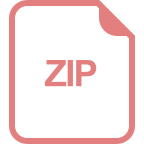
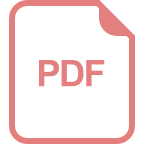
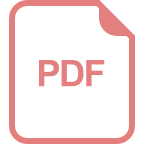