【实战演练】MATLAB优化货物配送路径的算法
发布时间: 2024-05-22 15:00:06 阅读量: 112 订阅数: 293 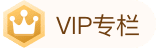
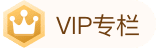
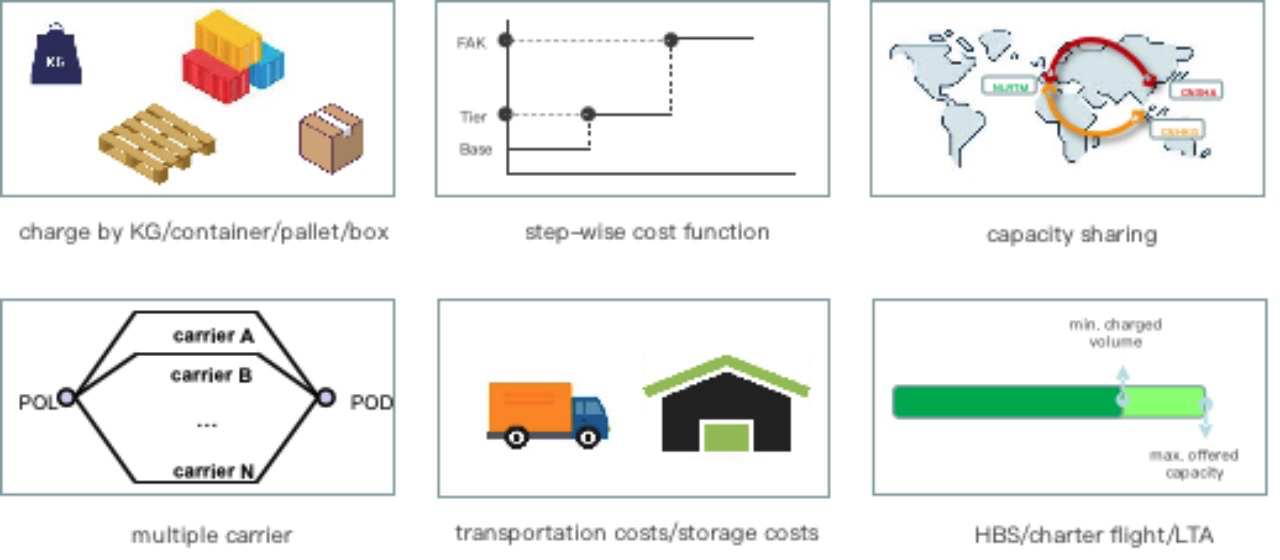
# 2.1 贪心算法
### 2.1.1 算法原理
贪心算法是一种启发式算法,它在每次决策时都选择当前看来最优的方案,而不考虑未来可能的后果。在货物配送路径优化中,贪心算法通常采用以下步骤:
1. **初始化:**将所有配送点标记为未访问。
2. **选择起点:**从所有未访问的配送点中选择一个作为起点。
3. **选择下一个配送点:**从起点出发,计算到所有未访问配送点的距离。选择距离最小的配送点作为下一个配送点。
4. **更新:**将下一个配送点标记为已访问,并更新起点到下一个配送点的距离。
5. **重复步骤 3 和 4:**直到所有配送点都被访问。
6. **返回:**返回访问配送点的顺序,即配送路径。
### 2.1.2 算法实现
MATLAB 中实现贪心算法的代码如下:
```matlab
function path = greedy_path(distances)
% 初始化
n = size(distances, 1);
visited = zeros(1, n);
path = zeros(1, n);
path(1) = 1;
visited(1) = 1;
% 循环选择下一个配送点
for i = 2:n
min_dist = inf;
next_node = 0;
for j = 1:n
if ~visited(j) && distances(path(i-1), j) < min_dist
min_dist = distances(path(i-1), j);
next_node = j;
end
end
path(i) = next_node;
visited(next_node) = 1;
end
end
```
# 2. 货物配送路径优化算法理论
### 2.1 贪心算法
#### 2.1.1 算法原理
贪心算法是一种启发式算法,它在每次决策时都选择当前看来最优的选项,而不考虑未来的影响。在货物配送路径优化中,贪心算法通常采用最近邻法,即在每个配送点选择距离当前配送点最近的未配送点作为下一个配送点。
#### 2.1.2 算法实现
```matlab
% 贪心算法实现
% 输入:配送点坐标,配送顺序
% 输出:最优配送路径
function path = greedy_algorithm(points, order)
% 初始化最优路径
path = [];
% 遍历配送点
for i = 1:length(order)
% 获取当前配送点
current_point = points(order(i), :);
% 计算当前配送点到未配送点的距离
distances = pdist2(current_point, points(order(i+1:end), :));
% 选择距离最近的未配送点
[~, idx] = min(distances);
next_point = order(i+idx);
% 更新最优路径
path = [path, next_point];
end
end
```
### 2.2 遗传算法
#### 2.2.1 算法原理
遗传算法是一种基于自然选择和遗传学原理的优化算法。在货物配送路径优化中,遗传算法将配送路径表示为染色体,并通过选择、交叉和变异等操作来进化染色体,从而寻找最优路径。
#### 2.2.2 算法实现
```matlab
% 遗传算法实现
% 输入:配送点坐标,种群大小,最大迭代次数
% 输出:最优配送路径
function path = genetic_algorithm(points, population_size, max_iterations)
% 初始化种群
population = generate_population(population_size, points);
% 进化种群
for i = 1:max_iterations
% 选择
parents = select_parents(population);
% 交叉
offspring = crossover(parents);
% 变异
offspring = mutate(offspring);
% 评估后代
offspring = evaluate_offspring(offspring, points);
% 更新种群
population = [population; offspring];
end
% 选择最优个体
best_individual = select_best_individual(population);
% 返回最优配送路径
path = best_individual.path;
end
```
### 2.3 粒子群算法
#### 2.3.1 算法原理
粒子群算法是一种基于群体智能的优化算法。在货物配送路径优化中,粒子群算法将配送路径表示为粒子,并通
0
0
相关推荐





